Removing Redundant Data from a DNG PA
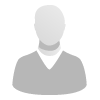
Accepted answer
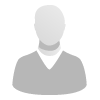
Hi Vaibhav,
If you set the URIs on the ReqIF data to match the URIs on the corresponding entities (data types, artifact definitions etc.) in the existing project then you would not get any duplicates when you do the import.
It may be quicker to roll back to the original project before the import, then set the URIs on the entities and then do the import again.
Otherwise you are left with no option but to manually delete all the attribute duplicates (plus you will have to synchronise the imported values between the duplicate attribute definitions) and then convert all the artifact types to be the same as the existing definition and then delete the duplicate definition.
Much easier to do the import again.
If you are coming from DOORS Classic then use the migration tool. It does much the synchronisation automatically and the process is much simpler than using ReqIF..
One other answer
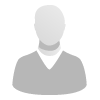
Hi,
I used below code for the same:
Main.java
package com.kpit.business;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.sql.Timestamp;
import java.util.ArrayList;
import java.util.List;
import java.util.Properties;
import java.util.logging.FileHandler;
import java.util.logging.Logger;
import java.util.logging.SimpleFormatter;
import org.apache.http.auth.UsernamePasswordCredentials;
//import org.apache.log4j.Logger;
import org.eclipse.lyo.client.exception.JazzAuthErrorException;
import org.eclipse.lyo.client.exception.JazzAuthFailedException;
import org.eclipse.lyo.client.exception.RootServicesException;
import org.eclipse.lyo.client.oslc.jazz.JazzFormAuthClient;
import org.eclipse.lyo.client.oslc.resources.Requirement;
import org.w3c.dom.DOMException;
import com.kpit.Pojo.Pojo;
import com.kpit.service.ReadArtifact;
import com.kpit.service.ReadDataTypes;
public class Main {
static Main main = new Main();
private String username;
private String password;
private String serverUrl;
private String projectArea;
private String artifactId;
private String attributeNameToBeChecked;
private String attributeName;
private String projectAreaUUId;
private String[] identifier = {"363", "365"};
//{"1382","3835","4686"};
//"1382","2851"};
// private String identifier;
/public void readConfig() throws IOException{
FileInputStream fis = new FileInputStream(new File("config.properties"));
Properties prop = new Properties();
prop.load(fis);
username = prop.getProperty("username").toString().trim();
password = prop.getProperty("password").toString().trim();
serverUrl = prop.getProperty("serverUrl").toString().trim();
projectArea = prop.getProperty("projectArea").toString().trim();
attributeName = prop.getProperty("attributeName").toString().trim();
projectAreaUUId = prop.getProperty("projectAreaUUId").toString().trim();
attributeNameToBeChecked = prop.getProperty("attributeNameToBeChecked").toString().trim();
}/
public void calls() throws DOMException, Exception{
Pojo pojo = new Pojo();
Logging frame=new Logging();
frame.setSize(300,100);
frame.setVisible(true);
}
public Main() {
System.out.println("Starting Execution ...");
}
public static void main(String[] args) throws DOMException, Exception {
try
{
try {
main.calls();
}
catch (RootServicesException | JazzAuthFailedException| JazzAuthErrorException e) {
e.printStackTrace();
}
}
catch (IOException e) {
e.printStackTrace();
}
}
}
Logging.java
package com.kpit.business;
import java.awt.BorderLayout;
import java.awt.Dimension;
import java.awt.GridLayout;
import java.awt.Toolkit;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import java.util.Properties;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JPasswordField;
import javax.swing.JTextField;
import javax.xml.parsers.ParserConfigurationException;
import org.apache.http.client.ClientProtocolException;
import org.eclipse.lyo.client.exception.JazzAuthErrorException;
import org.eclipse.lyo.client.exception.JazzAuthFailedException;
import org.eclipse.lyo.client.exception.RootServicesException;
import org.eclipse.lyo.client.oslc.resources.Requirement;
import org.w3c.dom.DOMException;
import org.xml.sax.SAXException;
import com.kpit.service.ReadArtifact;
public class Logging extends JFrame implements ActionListener{
private static String username;
private static String password;
private static String serverUrl;
private static String projectArea;
private static String artifactId;
private static String attributeNameToBeChecked;
private static String attributeName;
private static String projectAreaUUId;
private static String[] identifier = {"363", "365"};
//static Logging main = new Logging();
JButton SUBMIT;
static JPanel panel;
JLabel label1,label2;
JTextField text1;
JTextField text2;
static JFrame frame;
static Logging objCLI ;
public Logging()
{
frame = new JFrame("Login ");
panel = new JPanel();
label1 = new JLabel();
label1.setText("Username:");
text1 = new JTextField(15);
label2 = new JLabel();
label2.setText("Password:");
text2 = new JPasswordField(15);
SUBMIT=new JButton("SUBMIT");
panel=new JPanel(new GridLayout(3,1));
panel.add(label1);
panel.add(text1);
panel.add(label2);
panel.add(text2);
panel.add(SUBMIT);
add(panel,BorderLayout.CENTER);
SUBMIT.addActionListener(this);
setTitle("LOGIN FORM");
/ Dimension dim = Toolkit.getDefaultToolkit().getScreenSize();
frame.setLocation(dim.width / 2 - Logging.objCLI.getSize().width / 2, dim.height / 2 - Logging.objCLI.getSize().height / 2);
/
/ frame.add(panel);
frame.setSize(300, 100);
frame.setLocationRelativeTo(null);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
frame.dispose(); /
}
/ public static String methodSendCredential(String username, String password){
String credential = username + ";" + password;
return credential;
}/
public void actionPerformed(ActionEvent ae)
{
String username = text1.getText();
String password = text2.getText();
panel.setVisible(false);
panel.setEnabled(false);
frame.pack();
frame.dispose();
frame.setVisible(false);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
super.dispose();
List<Requirement> artifacts = new ArrayList<Requirement>();
try
{
Logging.readConfig();
}
catch (IOException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
try
{
ReadArtifact.createMappingFile(serverUrl, username, password, projectAreaUUId);
}
catch (ClientProtocolException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
} catch (RootServicesException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
} catch (JazzAuthFailedException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
} catch (JazzAuthErrorException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
} catch (IOException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
} catch (SAXException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
} catch (ParserConfigurationException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
ReadArtifact readArtifact ;//= new ReadArtifact();
// ReadDataTypes.readProjectDataTypes(username, password, serverUrl, projectArea);
for(int i=0; i<identifier.length; i++){
try {
artifacts = ReadArtifact.getDngArtifactXML(username, password, serverUrl, projectArea, attributeName, identifier[i], projectAreaUUId, attributeNameToBeChecked );
} catch (DOMException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
}
for (Requirement requirement : artifacts) {
System.out.println(requirement.getIdentifier());
}
// methodSendCredential(username, password);
/if (value1.equals("rvv6kor") && value2.equals("Fabvab@12345")) {
NextPage page=new NextPage();
page.setVisible(true);
JLabel label = new JLabel("Welcome:"+value1);
page.getContentPane().add(label);
}
else{
System.out.println("enter the valid username and password");
JOptionPane.showMessageDialog(this,"Incorrect login or password",
"Error",JOptionPane.ERROR_MESSAGE);
}/
}
public static void readConfig() throws IOException{
FileInputStream fis = new FileInputStream(new File("D:/dev/Executables/config.properties"));
Properties prop = new Properties();
prop.load(fis);
// username = prop.getProperty("username").toString().trim();
// password = prop.getProperty("password").toString().trim();
serverUrl = prop.getProperty("serverUrl").toString().trim();
projectArea = prop.getProperty("projectArea").toString().trim();
// attributeName = prop.getProperty("attributeName").toString().trim();
projectAreaUUId = prop.getProperty("projectAreaUUId").toString().trim();
attributeNameToBeChecked = prop.getProperty("attributeNameToBeChecked").toString().trim();
}
}
ReadArtifact.java
package com.kpit.service;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.PrintWriter;
import java.io.StringReader;
import java.io.StringWriter;
import java.net.URI;
import java.net.URISyntaxException;
import java.sql.Timestamp;
import java.util.ArrayList;
import java.util.Date;
import java.util.HashMap;
import java.util.Iterator;
import java.util.LinkedHashMap;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Properties;
import java.util.Set;
import java.util.logging.FileHandler;
import java.util.logging.Logger;
import java.util.logging.SimpleFormatter;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import javax.net.ssl.HostnameVerifier;
import javax.net.ssl.HttpsURLConnection;
import javax.xml.namespace.QName;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerException;
import javax.xml.transform.TransformerFactory;
import javax.xml.transform.dom.DOMSource;
import javax.xml.transform.stream.StreamResult;
import net.oauth.OAuthException;
import org.apache.http.Header;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.client.methods.HttpPut;
import org.apache.http.conn.scheme.Scheme;
import org.apache.http.conn.scheme.SchemeRegistry;
import org.apache.http.conn.ssl.SSLSocketFactory;
import org.apache.http.conn.ssl.X509HostnameVerifier;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.impl.conn.SingleClientConnManager;
import org.apache.http.util.EntityUtils;
//import org.apache.log4j.Logger;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.DataFormatter;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.apache.wink.client.ClientResponse;
import org.apache.xerces.parsers.DOMParser;
import org.eclipse.lyo.client.exception.JazzAuthErrorException;
import org.eclipse.lyo.client.exception.JazzAuthFailedException;
import org.eclipse.lyo.client.exception.ResourceNotFoundException;
import org.eclipse.lyo.client.exception.RootServicesException;
import org.eclipse.lyo.client.oslc.OSLCConstants;
import org.eclipse.lyo.client.oslc.OslcClient;
import org.eclipse.lyo.client.oslc.jazz.JazzFormAuthClient;
import org.eclipse.lyo.client.oslc.jazz.JazzRootServicesHelper;
import org.eclipse.lyo.client.oslc.resources.OslcQuery;
import org.eclipse.lyo.client.oslc.resources.OslcQueryParameters;
import org.eclipse.lyo.client.oslc.resources.OslcQueryResult;
import org.eclipse.lyo.client.oslc.resources.Requirement;
import org.eclipse.lyo.client.oslc.resources.RequirementCollection;
import org.eclipse.lyo.client.oslc.resources.RmConstants;
import org.eclipse.lyo.client.oslc.resources.RmUtil;
import org.eclipse.lyo.oslc4j.core.model.Link;
import org.eclipse.lyo.oslc4j.core.model.OslcMediaType;
import org.eclipse.lyo.oslc4j.core.model.ResourceShape;
import org.w3c.dom.DOMException;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.NamedNodeMap;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.InputSource;
import org.xml.sax.SAXException;
//import com.kpit.Pojo.Constants;
import com.kpit.business.CustomHttpClient;
public class ReadArtifact {
static String queryCapability;
static String serviceProviderUrl;
// static Logger logger = Logger.getLogger(ReadArtifact.class.getName());
static Logger logger;
static String etagArtifact;
static URI uri;
private static JazzRootServicesHelper rootServicesHelperCreateMappingFile = null;
private static JazzFormAuthClient clientCreateMappingFile = null;
private static JazzRootServicesHelper rootServicesHelper = null;
private static JazzFormAuthClient client = null;
private static JazzRootServicesHelper tempRootServicesHelper = null;
private static JazzFormAuthClient tempClient = null;
private static JazzRootServicesHelper rootServicesHelperCommitArtifact = null;
private static JazzFormAuthClient clientCommitArtifact = null;
private static JazzRootServicesHelper tempRootServicesHelperAttributeList = null;
private static JazzFormAuthClient tempClientAttributeList = null;
private static JazzRootServicesHelper putExecuteRootServicesHelper = null;
private static JazzFormAuthClient putExecuteClient = null;
static Map<String, String> mapAttributeList ;
private static String catalogUrl = null;
static Map<String, String> map = new HashMap<String, String>();
static Map<String, String> mapArtifactNameFromUri = new LinkedHashMap<String, String>();
// static String projectAreaUUId = null;
URI var;
private ReadArtifact(){
throw new RuntimeException();
}
/
Login to DNG
@return /
public static JazzFormAuthClient loginToDNG(String username, String password, String serverUrl, String projectArea) {
try{
rootServicesHelper = new JazzRootServicesHelper(serverUrl, OSLCConstants.OSLC_RM_V2);
client = rootServicesHelper.initFormClient(username, password);
client.formLogin();
System.out.println("rootServicesHelper "+rootServicesHelper);
System.out.println(client.formLogin());
System.out.println(username + password + serverUrl + projectArea);
//OslcMediaType.APPLICATION_RDF_XML);
catalogUrl= rootServicesHelper.getCatalogUrl();
if(client.formLogin()==200){
return client;
}
else
//throw new RuntimeException("Login Failed");
logger.info("----------------------------------------------------------------------------");
logger.info("Artifact Updated at: " + new Timestamp(System.currentTimeMillis()));
serviceProviderUrl = client.lookupServiceProviderUrl(rootServicesHelper.getCatalogUrl(), projectArea);
System.out.println(serviceProviderUrl);
queryCapability = client.lookupQueryCapability(serviceProviderUrl,OSLCConstants.OSLC_RM_V2,OSLCConstants.RM_REQUIREMENT_TYPE);
}
catch(RootServicesException e){
System.out.println(e.getMessage());
}
catch(JazzAuthFailedException e){
System.out.println(e.getMessage());
}
catch(JazzAuthErrorException e){
System.out.println(e.getMessage());
}
catch(ResourceNotFoundException e){
System.out.println(e.getMessage());
}
catch(IOException e){
System.out.println(e.getMessage());
}
catch(OAuthException e){
System.out.println(e.getMessage());
}
catch(URISyntaxException e){
System.out.println(e.getMessage());
}
return client;
}
/
* Check for duplicate Attributes
* @throws Exception
/
public static void artifactTypeKMap(String artifactType, String artifactIdentifierId, String typeOfArtifact,
String username, String password, String serverUrl, String projectArea, String projectAreaUUId, String attributeNameToBeChecked)
throws Exception
{
String artifactTypeName = null;
FileInputStream fis = new FileInputStream(new File("artifactTypeMappingFile.properties"));
Properties prop = new Properties();
prop.load(fis);
Set<Map.Entry<Object, Object>> set = prop.entrySet();
for (Entry<Object, Object> entry : set) {
System.out.println(entry.getKey() + " --- " + entry.getValue());
mapArtifactNameFromUri.put(entry.getValue()+"", entry.getKey()+"");
}
System.out.println(mapArtifactNameFromUri.size());
System.out.println(artifactType);
artifactType = artifactType.replaceAll(":", "@");
System.out.println(artifactType);
artifactTypeName = prop.getProperty(artifactType).toString().trim();
System.out.println("Artifact Type of Artifact is : " + artifactTypeName);
String copyArtifactTypeName = artifactTypeName;
// create regex to remove any numbers and special characters
artifactTypeName = artifactTypeName.trim();
Pattern pattern = Pattern.compile("[^0-9]");
Matcher matcher = pattern.matcher(artifactTypeName);
artifactTypeName = artifactTypeName.replaceAll("[\+\_\@\ \.\^:,]","");
artifactTypeName = artifactTypeName.replaceAll("[0-9]","");
System.out.println("Artifact Type after clean up is : " + artifactTypeName);
if(copyArtifactTypeName.equalsIgnoreCase(artifactTypeName)){
System.out.println("Artifact Type is not a duplicate");
}
else
{
// String uriForAtrifactTypeupdate = prop.getProperty("");
editArtifactType(copyArtifactTypeName, artifactTypeName, typeOfArtifact,copyArtifactTypeName,
mapArtifactNameFromUri.get(artifactTypeName), artifactIdentifierId, username, password,
serverUrl, projectArea, projectAreaUUId, attributeNameToBeChecked);
}
/for(int i=0; i<19; i++) {
if(artifactType.equalsIgnoreCase(artifactType+i)){
System.out.println("" + "");
// call artifact type change method
editArtifactType(artifactType+i, artifactType);
}
}*/
}
/
/
public static void setAttributeValues(Map<String, String> mapAttributueNameUrl){
}
/
* edit Artifact type, POST Artifact with new Attribute Values
* @throws Exception
/
public static void editArtifactType(String oldArtifactType,String newArtifactType,String artifactTypeName, String copyArtifactTypeName,
String dngIdUriInstanceShape, String artifactIdentifierId, String username, String password, String serverUrl,
String projectArea, String projectAreaUUId, String attributeNameToBeChecked) throws Exception{
// Map<String, String> mapAttributeList ;
System.out.println("Inside edit artifact type ");
ClientResponse response=null;
String resourceUri=null;
try{
rootServicesHelper = new JazzRootServicesHelper(serverUrl, OSLCConstants.OSLC_RM_V2);
client = rootServicesHelper.initFormClient(username, password);
client.formLogin();
if(client.formLogin()==200){
System.out.println("Logged in");
}
else {
System.out.println("Check the credentials .");
}
tempRootServicesHelper = new JazzRootServicesHelper(serverUrl, OSLCConstants.OSLC_RM_V2);
tempClient = rootServicesHelper.initFormClient(username, password);
tempClient.formLogin();
tempRootServicesHelperAttributeList = new JazzRootServicesHelper(serverUrl, OSLCConstants.OSLC_RM_V2);
tempClientAttributeList = tempRootServicesHelperAttributeList.initFormClient(username, password);
tempClientAttributeList.formLogin();
putExecuteRootServicesHelper = new JazzRootServicesHelper(serverUrl, OSLCConstants.OSLC_RM_V2);
putExecuteClient = putExecuteRootServicesHelper.initFormClient(username, password);
putExecuteClient.formLogin();
mapAttributeList = createAttributeList(serverUrl, projectAreaUUId);
catalogUrl= rootServicesHelper.getCatalogUrl();
System.out.println(catalogUrl);
//throw new RuntimeException("Login Failed");
serviceProviderUrl = client.lookupServiceProviderUrl(catalogUrl, projectArea);
System.out.println("serviceProviderUrl : "+serviceProviderUrl);
// System.out.println(rootServicesHelper + " &&& " + client);
String queryCapabilityUrl= client.lookupQueryCapability(serviceProviderUrl, OSLCConstants.OSLC_RM_V2, OSLCConstants.RM_REQUIREMENT_TYPE);
System.out.println("queryCapabilityUrl : "+queryCapabilityUrl);
String requirementFactory = client.lookupCreationFactory(serviceProviderUrl, OSLCConstants.OSLC_RM_V2, OSLCConstants.RM_REQUIREMENT_TYPE);
System.out.println("requirementFactory : "+ requirementFactory);
// Service provider URL maintained as per component or baseline requirements
OslcQueryParameters queryParams = new OslcQueryParameters();
String identifier = "363";
queryParams.setPrefix("dcterms=<http://purl.org/dc/terms/>");
queryParams.setWhere("dcterms:identifier="+ artifactIdentifierId);
queryParams.setSelect("dcterms:title");
System.out.println("artifactIdentifierId "+ artifactIdentifierId);
OslcQuery query = new OslcQuery(client, queryCapabilityUrl, 15, queryParams);
OslcQueryResult result = query.submit();
System.out.println("Query Running .... ");
String[] urls= result.getMembersUrls();
// projectAreaUUId = urls[0].substring(urls[0].indexOf("resources")+10, urls[0].length());
// System.out.println("projectAreaUUId : "+ projectAreaUUId);
String temp = dngIdUriInstanceShape;
Set<Map.Entry<String, String>> set = mapArtifactNameFromUri.entrySet();
HashMap<String, String> reverseMap = new HashMap<String, String>();
for (Entry<String, String> entry : set) {
reverseMap.put(entry.getValue(),entry.getKey());
}
// System.out.println("reverse map : " + reverseMap.get(temp));
if(artifactTypeName.equalsIgnoreCase("http://open-services.net/ns/rm#Requirement") ||
artifactTypeName.equalsIgnoreCase("http://jazz.net/ns/rm#Text")){
System.out.println("Updating an Artifact ... ");
Requirement requirementArtifactToBeUpdated = null;
for(String s:urls)
{
requirementArtifactToBeUpdated = null;
resourceUri=(String)s;
System.out.println("Artifact URL is : "+ resourceUri);
response = client.getResource(resourceUri , OslcMediaType.APPLICATION_RDF_XML);
requirementArtifactToBeUpdated = response.getEntity(Requirement.class);
System.out.println("requirementArtifactToBeUpdated :"+requirementArtifactToBeUpdated.getIdentifier());
System.out.println("dngIdUriInstanceShape : "+dngIdUriInstanceShape);
String tempForDataTypes = dngIdUriInstanceShape;
dngIdUriInstanceShape = dngIdUriInstanceShape.replaceAll("@", ":");
System.out.println("dngIdUriInstanceShape : "+dngIdUriInstanceShape);
uri = new URI(dngIdUriInstanceShape);
System.out.println("uri -- "+uri);
System.out.println("Original type of Artifact is : -- "+requirementArtifactToBeUpdated.getInstanceShape());
requirementArtifactToBeUpdated.setInstanceShape(uri);
etagArtifact= response.getHeaders().getFirst(OSLCConstants.ETAG);
System.out.println("etagArtifact2 : " + etagArtifact);
System.out.println("now setting type to : --- " + uri );
// System.out.println("setting the modidfied date as ----> "+ requirementArtifactToBeUpdated.getModified());
// requirementArtifactToBeUpdated.setModified(new Date(System.currentTimeMillis()));
// requirementArtifactToBeUpdated.setDescription(requirementArtifactToBeUpdated.getDescription() + "1");
// requirementArtifactToBeUpdated.setDescription("description from code");
requirementArtifactToBeUpdated.setTitle("title from code"+ new Date(System.currentTimeMillis()));
// requirementArtifactToBeUpdated.set
// --------------------------------------
// url for component https://hjalmdclm.kpit.com:9443/rm/cm/component/cqIf4MFnEeitSbv70zI1XA
// CHECKING FOR DUPLICATE ATTRIBUTES INSIDE THIS PARTICULAR ARTIFACT
// requirementArtifactToBeUpdated.getAbout();
Map<QName, Object> mapGetExtendedProperties = requirementArtifactToBeUpdated.getExtendedProperties();
Map<QName, Object> mapSetExtendedProperties = mapGetExtendedProperties;
String strTemp = null;
Set<Map.Entry<QName, Object>> entrySet = mapGetExtendedProperties.entrySet();
int z=0;
Map<String, String> mapAttributueNameUrl = new HashMap<String,String>();
// USING CONTINUE TAG BLOCK TO BREAK FROM NESTED LOOPS IF ATTRIBUTE NAME IS NOT
// WHAT IS ASKED FOR FROM USER
search:
for (Map.Entry<QName, Object> entry : entrySet) {
if(true)
{
// Attribute value to be checked and replaced for duplicate attributes
// Gets name of attribute
System.out.println(entry.getKey());
String artifactAttributeName = entry.getKey()+"";
artifactAttributeName = artifactAttributeName.replace("{", "");
artifactAttributeName = artifactAttributeName.replace("}", "");
System.out.println("artifactAttributeName after cleanup of braces : " + artifactAttributeName);
// Getting attribute name from the url to set its value using QMap.
String urlAttributeName = artifactAttributeName;
String attributeName = null;
if(!(urlAttributeName.contains("types"))){
continue;
}
else{
HttpGet connGetAttribute = new HttpGet(urlAttributeName);
connGetAttribute.setHeader("Content-Type","application/rdf+xml");
connGetAttribute.setHeader("Accept", "application/rdf+xml");
HttpResponse httpResponse = client.getHttpClient().execute(connGetAttribute);
System.out.println(httpResponse.getStatusLine().getStatusCode());
InputStream inputStream = httpResponse.getEntity().getContent();
System.out.println(inputStream);
DocumentBuilderFactory dbFactory = DocumentBuilderFactory.newInstance();
DocumentBuilder dBuilder = dbFactory.newDocumentBuilder();
Document document = dBuilder.parse(inputStream);
NodeList element = document.getElementsByTagName("rdfs:label");
System.out.println(element);
for (int p = 0; p < element.getLength(); p++) {
attributeName = element.item(p).getTextContent();
System.out.println(attributeName);
break;
}
// z = z+1;
String attributeNameValueUrl = entry.getValue()+"";
String tempAttributeNameValueUrl = attributeNameValueUrl;
// THE MAP FOR EACH ATTRIBUTE WITH NAME AND VALUE URL OF TYPE STRING AND TEXT
if(attributeNameValueUrl.contains("https") || attributeNameValueUrl.contains("http")){
if(!(mapAttributueNameUrl.containsKey(attributeName))){
if(entry.getKey() != null ){
strTemp = attributeName;
Pattern pattern = Pattern.compile("[^0-9]");
Matcher matcher = pattern.matcher(strTemp);
strTemp = strTemp.replaceAll("[\+\@\.\^:,]","");
strTemp = strTemp.replaceAll("[\:,]"," ");
strTemp = strTemp.replaceAll("[0-9]","");
System.out.println("attributeName After cleanup of number duplicates : "+ strTemp);
strTemp = strTemp.trim();
if(strTemp.equalsIgnoreCase(attributeNameToBeChecked))
//(attributeName.equalsIgnoreCase(strTemp) && attributeName.equalsIgnoreCase(attributeNameToBeChecked)) || (attributeName.equalsIgnoreCase(attributeNameToBeChecked)) )
{
continue search;
}
else if(attributeName.equals(strTemp)){
mapAttributueNameUrl.put(attributeName, tempAttributeNameValueUrl);
}
else {
System.out.println("its a duplicate attribute name. commit the original");
// if Status 2 is the first value . we need to read the value of Status from web
// as it wont be available in map.
// or we can update with post using refined attribute name for post
String valueToBeUpdated = tempAttributeNameValueUrl;
// creating artifact stream on which values will be updated
HttpGet tempConnGetAttribute = new HttpGet(urls[0]);
tempConnGetAttribute.setHeader("Content-Type","application/rdf+xml");
tempConnGetAttribute.setHeader("Accept", "application/rdf+xml");
HttpResponse tempHttpResponse = tempClient.getHttpClient().execute(tempConnGetAttribute);
System.out.println(tempHttpResponse.getStatusLine().getStatusCode());
InputStream tempInputStream = tempHttpResponse.getEntity().getContent();
System.out.println("tempInputStream "+tempInputStream);
System.out.println("valueToBeUpdated : "+valueToBeUpdated);
System.out.println("urls : " + urls[0]);
int q=0;
logger.info("Updating Artifact " + requirementArtifactToBeUpdated.getIdentifier()
+ "'s Duplicate Atribute " + strTemp );
String xmlString = updatingResponse(tempInputStream, urls, valueToBeUpdated,strTemp, q);
StringEntity stringEntity =new StringEntity(xmlString,"UTF-8");
HttpClient httpclient= new DefaultHttpClient();
//CustomHttpClient httpclient= new CustomHttpClient();
// Generating ETAG/etag Value for PUT
HttpGet httpGet=new HttpGet(urls[0]);
httpGet.addHeader("Content-Type","application/rdf+xml");
httpGet.addHeader("Accept", "application/rdf+xml");
HttpResponse getResponse = OslcUtils.sendGetForSecureDocument(serverUrl, httpGet, username, password, httpclient);
String etag= OslcUtils.getETag(getResponse);
System.out.println("etag ::: "+etag);
// String etag = response.getHeaders().getFirst(OSLCConstants.ETAG);
HttpEntity e = getResponse.getEntity();
e.consumeContent();
HttpPut httpPut= new HttpPut(urls[0]);
System.out.println(urls[0]);
System.out.println(resourceUri);
httpPut.setEntity(stringEntity);
httpPut.addHeader("Content-Type","application/rdf+xml");
httpPut.addHeader("Accept", "application/rdf+xml");
// httpPut.addHeader(Constants.DOORSRP_REQUEST_TYPE, Constants.DOORSRP_REQUEST_TYPE_VALUE);
httpPut.setHeader("If-Match", etag);
HttpClient httpclientPut= new DefaultHttpClient();
HttpResponse responsePut = httpclient.execute(httpPut);
System.out.println("x : "+ responsePut.getStatusLine().getStatusCode());
/*Header[] hearde = responsePut.getAllHeaders();
for (Header header : hearde) {
System.out.println(" " + header.getName());
System.out.println(" " + header.getValue());
}*/
HttpEntity en1 = responsePut.getEntity();
EntityUtils.consume(en1);
// post value from attributeName to strTemp attribute
// use POST or QName
// post null to duplicate attribute also i.e attributeName var
}
}
}
}
else{
// put map for string attribute vales above is for enum types
//set vqalue for syring types attributes here
mapAttributueNameUrl.put(attributeName, entry.getValue()+"");
String valueToBeUpdated = tempAttributeNameValueUrl;
// if(!(mapAttributueNameUrl.containsKey(attributeName))){
if(entry.getKey() != null ){
String strTemp2 = attributeName;
Pattern pattern = Pattern.compile("[^0-9]");
Matcher matcher = pattern.matcher(strTemp2);
strTemp2 = strTemp2.replaceAll("[\+\@\.\^:,]","");
strTemp2 = strTemp2.replaceAll("[\_:,]"," ");
strTemp2 = strTemp2.replaceAll("[0-9]","");
System.out.println("attributeName After cleanup of number duplicates : "+ strTemp2);
strTemp2 = strTemp2.trim();
if(strTemp2.equalsIgnoreCase(attributeNameToBeChecked)){
continue search;
}
else if(attributeName.equals(strTemp2)){
mapAttributueNameUrl.put(attributeName, tempAttributeNameValueUrl);
}
else{
// creating artifact stream on which values will be updated
HttpGet tempConnGetAttribute = new HttpGet(urls[0]);
tempConnGetAttribute.setHeader("Content-Type","application/rdf+xml");
tempConnGetAttribute.setHeader("Accept", "application/rdf+xml");
HttpResponse tempHttpResponse = tempClient.getHttpClient().execute(tempConnGetAttribute);
System.out.println(tempHttpResponse.getStatusLine().getStatusCode());
InputStream tempInputStream = tempHttpResponse.getEntity().getContent();
System.out.println("tempInputStream "+tempInputStream);
System.out.println("valueToBeUpdated : "+valueToBeUpdated);
System.out.println("urls : " + urls[0]);
int q=1;
logger.info("Updating Artifact " + requirementArtifactToBeUpdated.getIdentifier()
+ "'s Duplicate Atribute " + strTemp2 );
String xmlString = updatingResponse(tempInputStream, urls, valueToBeUpdated,strTemp2, q);
StringEntity stringEntity = new StringEntity(xmlString,"UTF-8");
// CustomHttpClient httpclient= new CustomHttpClient();
HttpClient httpclient= new DefaultHttpClient();
// Generating ETAG/etag Value for PUT
HttpGet httpGet=new HttpGet(urls[0]);
httpGet.addHeader("Content-Type","application/rdf+xml");
httpGet.addHeader("Accept", "application/rdf+xml");
HttpResponse getResponse = OslcUtils.sendGetForSecureDocument(serverUrl, httpGet, username, password, httpclient);
System.out.println("etag code : "+getResponse.getStatusLine().getStatusCode());
System.out.println("etag code : "+getResponse.getStatusLine());
String etag= OslcUtils.getETag(getResponse);
System.out.println("%220%22&%22_ZrnlDm15EemFI_UzVjMASQ%22 "+etag);
// etag = etag.substring(12, etag.length()-4);
System.out.println("etag ::: "+etag);
// String etag = response.getHeaders().getFirst(OSLCConstants.ETAG);
HttpEntity e = getResponse.getEntity();
e.consumeContent();
HttpPut httpPut= new HttpPut(urls[0]);
httpPut.setEntity(stringEntity);
httpPut.setHeader("Content-Type","application/rdf+xml");
httpPut.setHeader("Accept", "application/rdf+xml");
httpPut.setHeader("If-Match", etag);
// httpPut.addHeader(Constants.DOORSRP_REQUEST_TYPE, Constants.DOORSRP_REQUEST_TYPE_VALUE);
HttpClient httpclientPut= new DefaultHttpClient();
HttpResponse responsePut = httpclient.execute(httpPut);
System.out.println("Put Response : "+responsePut.getStatusLine().getStatusCode());
Header[] hearde = responsePut.getAllHeaders();
for (Header header : hearde) {
System.out.println(" " + header.getName());
System.out.println(" " + header.getValue());
}
// String newPath = null;
/*if(responsePut.getStatusLine().getStatusCode() == 302){
Header[] hearde = responsePut.getAllHeaders();
for (Header header : hearde) {
System.out.println(" " + header.getName());
if(header.getName().equalsIgnoreCase("location")){
System.out.println(" " + header.getValue());
newPath = header.getValue();
break;
}
}
//System.exit(1);
}
HttpPut httpPut2= new HttpPut(newPath);
httpPut2.setEntity(stringEntity);
httpPut2.setHeader("Content-Type","application/rdf+xml");
httpPut2.setHeader("Accept", "application/rdf+xml");
httpPut2.setHeader("If-Match", etag);
HttpClient httpclientPutNewLocal= new DefaultHttpClient();
HttpResponse responsePutNewLocal = httpclientPutNewLocal.execute(httpPut2);
System.out.println("Put Response : "+responsePutNewLocal.getStatusLine().getStatusCode());
/
HttpEntity en2 = responsePut.getEntity();
EntityUtils.consume(en2);
HttpEntity en3 = httpResponse.getEntity();
EntityUtils.consume(en3);
HttpEntity en4 = tempHttpResponse.getEntity();
EntityUtils.consume(en4);
/
StringEntity stringEntity =new StringEntity(xmlString,"UTF-8");
HttpPut httpPut= new HttpPut(urls[0]);
httpPut.setEntity(stringEntity);
// Generating ETAG/etag Value for PUT
HttpGet httpGet=new HttpGet(urls[0]);
httpGet.setHeader("Content-Type","application/rdf+xml");
httpGet.setHeader("Accept", "application/rdf+xml");
HttpClient httpclient= new DefaultHttpClient();
HttpResponse getResponse = putExecuteClient.getHttpClient().execute(httpGet);
//OslcUtils.sendGetForSecureDocument(serverUrl, httpGet, username, password, httpclient);
String etag= OslcUtils.getETag(getResponse);
System.out.println("etag ::: "+etag);
httpPut.setHeader("Content-Type","application/rdf+xml");
httpPut.setHeader("Accept", "application/rdf+xml");
httpPut.addHeader("If-Match", etag);
// httpPut.addHeader(Constants.DOORSRP_REQUEST_TYPE, Constants.DOORSRP_REQUEST_TYPE_VALUE);
// httpPut.addHeader(Constants.IF_MATCH, etag);
HttpResponse getResponse = OslcUtils.sendGetForSecureDocument(serverURL, httpGet, username, password, httpclient);
String etag= OslcUtils.getETag(getResponse);
try{
HttpResponse responsePut = httpclient.execute(httpPut);
}
catch(Exception e){
e.printStackTrace();
}/
}
}
}
// }
// QName qname = new QName(arg0, arg1, arg2);
System.out.println(""+entry.getKey().getLocalPart());
System.out.println(""+entry.getKey().getNamespaceURI());
System.out.println(""+entry.getKey().getPrefix());
System.out.println(""+entry.getValue());
System.out.println("---------------------------");
System.out.println();
}
}
}
// }
Set<Map.Entry<String,String>> entrySet4 = mapAttributueNameUrl.entrySet();
for (Entry<String, String> entry2 : entrySet4) {
System.out.println("..................");
System.out.println(entry2.getKey());
System.out.println(entry2.getValue());
System.out.println("..................");
}
// --------------------------------------
/// CHECKING FOR DUPLICATE DATA TYPES INSIDE THIS PARTICULAR ARTIFACT /
// SAVING THE RESOURCE ARTIFACT
// String etag = response.getHeaders().getFirst(OSLCConstants.ETAG);
System.out.println("Update Resource URL --- "+ s);
response.consumeContent();
rootServicesHelperCommitArtifact = new JazzRootServicesHelper(serverUrl, OSLCConstants.OSLC_RM_V2);
clientCommitArtifact = rootServicesHelperCommitArtifact.initFormClient(username, password);
clientCommitArtifact.formLogin();
ClientResponse responsecommitArtifact = clientCommitArtifact.getResource(resourceUri , OslcMediaType.APPLICATION_RDF_XML);
etagArtifact = responsecommitArtifact.getHeaders().getFirst(OSLCConstants.ETAG);
System.out.println("etagArtifact : " + etagArtifact);
try{
Requirement req = responsecommitArtifact.getEntity(Requirement.class);
req.setInstanceShape(uri);
req.setTitle("title from code"+ new Date(System.currentTimeMillis()));
ClientResponse getResult = client.updateResource(s, (Object)req, OslcMediaType.APPLICATION_RDF_XML, OslcMediaType.APPLICATION_RDF_XML, etagArtifact);
//(requirementArtifactToBeUpdated.getIdentifier(), requirementArtifactToBeUpdated, OSLCConstants.CT_RDF);
System.out.println("etagArtifact : " + etagArtifact);
System.out.println("Status of update : "+getResult.getStatusCode() +" --- "+ getResult.getMessage());
String tempUriForLogger = uri +"";
tempUriForLogger = tempUriForLogger.replace(":", "@");
logger.info("Updating Artifact " + req.getIdentifier() + "'s Duplicate Artifact Type to " + reverseMap.get(tempUriForLogger));
logger.info("Artifact " + req.getIdentifier() + " updated");
System.out.println("Artifact " + req + "Attribute has been updated to " + reverseMap.get(tempUriForLogger) );
getResult.consumeContent();
//logger.info("Execution completed at " + new Timestamp(System.currentTimeMillis()) );
}
catch(Exception ex){
throw ex;
// System.out.println("Error while updating the Artifact : "+ex);
// logger.info("Please enter a valid ID");
}
break;
}
}
else if(artifactTypeName.equalsIgnoreCase("http://open-services.net/ns/rm#RequirementCollection")||
artifactTypeName.equalsIgnoreCase("http://jazz.net/ns/rm#Module")) {
System.out.println("Modules can not be Cleaned and Updated in this Version. Please wait for next Release");
RequirementCollection requirementArtifactToBeUpdated = null;
/for(String s:urls)
{
requirementArtifactToBeUpdated = null;
resourceUri=(String)s;
response = client.getResource(resourceUri , OslcMediaType.APPLICATION_RDF_XML);
requirementArtifactToBeUpdated = response.getEntity(RequirementCollection.class);
System.out.println("requirementArtifactToBeUpdated :"+requirementArtifactToBeUpdated.getIdentifier());
System.out.println("dngIdUriInstanceShape : "+dngIdUriInstanceShape);
// String temp = dngIdUriInstanceShape;
dngIdUriInstanceShape = dngIdUriInstanceShape.replaceAll("@", ":");
System.out.println("dngIdUriInstanceShape : "+dngIdUriInstanceShape);
URI uri = new URI(dngIdUriInstanceShape);
System.out.println("uri -- "+uri);
System.out.println("Original type of Artifact is : -- "+requirementArtifactToBeUpdated.getInstanceShape());
// getting artifact type name from instance shape uri
ClientResponse clientResponseAttribute = client.getResource(dngIdUriInstanceShape, OSLCConstants.CT_RDF);
ResourceShape resourceShape = clientResponseAttribute.getEntity(ResourceShape.class);
System.out.println("Name of new Artifact type dwrived from uri si :"+resourceShape.getAbout());
Set<Map.Entry<String, String>> set = mapArtifactNameFromUri.entrySet();
HashMap<String, String> reverseMap = new HashMap<String, String>();
for (Entry<String, String> entry : set) {
reverseMap.put(entry.getValue(),entry.getKey());
}
System.out.println("reverse map : " + reverseMap.get(temp));
ResourceShape featureInstanceShape = RmUtil.lookupRequirementsInstanceShapes((String)serviceProviderUrl,
OSLCConstants.OSLC_RM_V2, OSLCConstants.RM_REQUIREMENT_COLLECTION_TYPE,
//(String)"http://open-services.net/ns/rm#", (String)"http://open-services.net/ns/rm#RequirementCollection",
(OslcClient)client, "Use Case Specification");
//reverseMap.get(temp)) ;
// "/DeletedDataMigration/Lithium Module - Module");
System.out.println("featureInstanceShape -- : " + featureInstanceShape.getTitle() + "........ " + featureInstanceShape.getAbout());
requirementArtifactToBeUpdated.setInstanceShape(featureInstanceShape.getAbout());
// requirementArtifactToBeUpdated.setInstanceShape(uri);
System.out.println("now setting type to : --- " + uri );
// System.out.println("setting the modidfied date as ----> "+ requirementArtifactToBeUpdated.getModified());
// requirementArtifactToBeUpdated.setModified(new Date(System.currentTimeMillis()));
// setting the modidfied date as ----> Fri Dec 14 12:08:40 IST 2018
// requirementArtifactToBeUpdated.setDescription(requirementArtifactToBeUpdated.getDescription()+ " " +new Timestamp(System.currentTimeMillis()));
// requirementArtifactToBeUpdated.setTitle("title from code");
String etag = response.getHeaders().getFirst(OSLCConstants.ETAG);
try{
System.out.println("Update Resource URL --- "+ s);
ClientResponse getResult = client.updateResource(s, (Object)requirementArtifactToBeUpdated, OslcMediaType.APPLICATION_RDF_XML, OslcMediaType.APPLICATION_RDF_XML, etag);
//(requirementArtifactToBeUpdated.getIdentifier(), requirementArtifactToBeUpdated, OSLCConstants.CT_RDF);
System.out.println("Status of update : "+getResult.getStatusCode() +" --- "+ getResult.getMessage());
System.out.println("Artifact " + requirementArtifactToBeUpdated + "Attribute has been updated to " + mapArtifactNameFromUri.get(requirementArtifactToBeUpdated.getInstanceShape()) );
getResult.consumeContent();
}
catch(Exception ex){
throw ex;
// System.out.println("Error while updating the Artifact : "+ex);
// logger.info("Please enter a valid ID");
}
break;
}/
}
// moduleArtifactToBeUpdated.setInstanceShape(new URI(""));
}
catch(Exception ex){
throw ex;
}
// post the edited xml for requirement artifact with new artifact type
// Set<String> setDuplicateArtifactTypes = new LinkedHashSet<String>();
// deleteDuplicateArtifactTypes(setDuplicateArtifactTypes);
}
public static void createMappingFile(String serverUrl, String username, String password, String projectAreaUUid) throws ClientProtocolException, IOException, SAXException, ParserConfigurationException, RootServicesException, JazzAuthFailedException, JazzAuthErrorException{
boolean append = true;
FileHandler handler = new FileHandler("./logfile.log", append);
SimpleFormatter formatter = new SimpleFormatter();
handler.setFormatter(formatter);
logger = Logger.getLogger(ReadArtifact.class.getName());
logger.setUseParentHandlers(false);
logger.addHandler(handler);
logger.info("---------------------------------");
logger.info("Execution started at " + new Timestamp(System.currentTimeMillis()) + " by " + username);
PrintWriter pwrt = new PrintWriter("artifactTypeMappingFile.properties","UTF-8");
String url = serverUrl + "/types?resourceContext=" + serverUrl + "/process/project-areas/" + projectAreaUUid;
//
url = url.trim();
rootServicesHelperCreateMappingFile = new JazzRootServicesHelper(serverUrl, OSLCConstants.OSLC_RM_V2);
clientCreateMappingFile = rootServicesHelperCreateMappingFile.initFormClient(username, password);
clientCreateMappingFile.formLogin();
HttpPost connPost = new HttpPost();
HttpGet conn = new HttpGet(url);
conn.setHeader("Content-Type","application/rdf+xml");
conn.setHeader("Accept", "application/rdf+xml");
// conn.setHeader("OSLC-Core-Version", "2.0");
// conn.setHeader("vvc.configuration", "");
// conn.addHeader("Accept-Encoding", "application/x-www-form-urlencoded;charset=UTF-8");
// UUID uid = UUID
List<String> list = new ArrayList<String>();
HttpResponse httpResponseAttributeList = clientCreateMappingFile.getHttpClient().execute(conn);
System.out.println(httpResponseAttributeList.getStatusLine().getStatusCode());
InputStream inputStreamAttributeList = httpResponseAttributeList.getEntity().getContent();
DocumentBuilderFactory dbFactoryCreateMappingFile = DocumentBuilderFactory.newInstance();
DocumentBuilder dBuilderCreateMappingFile = dbFactoryCreateMappingFile.newDocumentBuilder();
Document documentCreateMappingFile = dBuilderCreateMappingFile.parse(inputStreamAttributeList);
NodeList nodeListCreateMappingFile = documentCreateMappingFile.getElementsByTagName("rm:ObjectType");
for (int z = 0; z < nodeListCreateMappingFile.getLength(); z++) {
Node nodeCreateMapingFileArtifactUrl = nodeListCreateMappingFile.item(z);
Element elementCreateMapingFileArtifactUrl = (Element) nodeCreateMapingFileArtifactUrl;
String artifactTypeUrlString = elementCreateMapingFileArtifactUrl.getAttribute("rdf:about");
System.out.println("artifactTypeeNameString : " + artifactTypeUrlString);
NodeList childNodeListCreateMappingFile = nodeListCreateMappingFile.item(z).getChildNodes();
String artifactTypeNameString = null;
for(int r=0; r<childNodeListCreateMappingFile.getLength(); r++)
{
if(childNodeListCreateMappingFile.item(r).getNodeName().equals("dcterms:title")){
Node nodeCreateMappingFileArtifactName = childNodeListCreateMappingFile.item(r);
Element elementCreateMappingFileArtifactName = (Element) nodeCreateMappingFileArtifactName;
artifactTypeNameString = elementCreateMappingFileArtifactName.getTextContent();
System.out.println("artifactTypeeNameString " + elementCreateMappingFileArtifactName.getTextContent());
}
}
artifactTypeUrlString = artifactTypeUrlString.replace(':', '@');
pwrt.write(artifactTypeUrlString + " = " + artifactTypeNameString);
pwrt.write("\n");
System.out.println("-----------------------");
}
pwrt.close();
}
public static Map<String, String> createAttributeList(String serverurl, String projectAreaUUId) throws ClientProtocolException, IOException,
SAXException, ParserConfigurationException{
Map<String, String> mapAttributeList = new HashMap<String, String>();
String url = serverurl + "/types?resourceContext=" + serverurl + "/process/project-areas/" + projectAreaUUId;
url = url.trim();
HttpPost connPost = new HttpPost();
HttpGet conn = new HttpGet(url);
conn.setHeader("Content-Type","application/rdf+xml");
conn.setHeader("Accept", "application/rdf+xml");
// conn.setHeader("OSLC-Core-Version", "2.0");
// conn.setHeader("vvc.configuration", "");
// conn.addHeader("Accept-Encoding", "application/x-www-form-urlencoded;charset=UTF-8");
// UUID uid = UUID
List<String> list = new ArrayList<String>();
HttpResponse httpResponseAttributrList = client.getHttpClient().execute(conn);
HttpResponse httpResponseAttributeList = tempClientAttributeList.getHttpClient().execute(conn);
System.out.println(httpResponseAttributrList.getStatusLine().getStatusCode());
System.out.println(httpResponseAttributeList.getStatusLine().getStatusCode());
InputStream inputStream = httpResponseAttributrList.getEntity().getContent();
System.out.println(inputStream);
InputStream inputStreamAttributeList = httpResponseAttributeList.getEntity().getContent();
/ PRINT INUT STREAM RESPONSE
InputStreamReader isr = new InputStreamReader(inputStream);
BufferedReader reader = new BufferedReader(isr);
StringBuilder out =new StringBuilder();
String line;
while((line = reader.readLine()) != null) {
out.append(line);
out.append('\n');
}
System.out.println(out.toString());
/
String line = null;
BufferedReader br = new BufferedReader(new InputStreamReader(inputStream,"UTF8"));
StringBuilder sb = new StringBuilder();
while((line = br.readLine()) != null){
sb.append(line);
}
if(br != null){
br.close();
}
System.out.println(line + sb);
DOMParser parser = new DOMParser();
parser.parse(new InputSource(new StringReader(sb.toString())));
Document document = parser.getDocument();
NodeList childNodes = document.getDocumentElement().getChildNodes();
// NodeList childNodesDataTypesList = childNodes;
/ DocumentBuilderFactory dbFactoryAttributeList = DocumentBuilderFactory.newInstance();
DocumentBuilder dBuilderAttributeList = dbFactoryAttributeList.newDocumentBuilder();
Document documentAttributeList = dBuilderAttributeList.parse(inputStreamAttributeList);
NodeList elementAttributeList = documentAttributeList.getElementsByTagName("rm:AttributeDefinition");
System.out.println(elementAttributeList);
String attributeName = null;
for (int z = 0; z < elementAttributeList.getLength(); z++) {
attributeName = elementAttributeList.item(z).getTextContent();
System.out.println(elementAttributeList.item(z).getTextContent() );
System.out.println(elementAttributeList.item(z).getLocalName());
System.out.println(elementAttributeList.item(z).getLocalName());
System.out.println(elementAttributeList.item(z).getNamespaceURI());
System.out.println(attributeName);
System.out.println("-----------------------");
break;
}/
for(int i=0; i < childNodes.getLength() ; i++){
int t=i;
int p=0;
// System.out.println("childNodes.item(i).getNodeName() : " + childNodes.item(i).getNodeName());
// just save the urls with names. dont bother abt enum values, just need the attribute url
// value is handled in method as toBeUpdated variable
if("rm:AttributeDefinition".equals(childNodes.item(i).getNodeName())){
NodeList suchChildNodes = childNodes.item(i).getChildNodes();
/System.out.println("Matching Child Nodes -- "+childNodes.item(i).getNodeName());
System.out.println("Matching Child Nodes -- "+childNodes.item(i).getTextContent());
System.out.println("Matching Child Nodes -- "+childNodes.item(i).getLocalName());
System.out.println("Matching Child Nodes -- "+childNodes.item(i).getBaseURI());
System.out.println("Matching Child Nodes -- "+childNodes.item(i).getNodeValue());
System.out.println("Matching Child Nodes -- "+childNodes.item(i).getNamespaceURI());
System.out.println("Matching Child Nodes -- "+childNodes.item(i).getNodeType());
System.out.println("Matching Child Nodes -- "+childNodes.item(i).getAttributes().getNamedItem("rdf:about"));
/
for(int j=0; j < suchChildNodes.getLength(); j++){
// System.out.println("suchChildNodes.item(j).getNodeName() : "+suchChildNodes.item(j).getNodeName());
if("dcterms:title".equalsIgnoreCase(suchChildNodes.item(j).getNodeName()) ){
/System.out.println("suchChildNodes.item(j).getTextContent() "+suchChildNodes.item(j).getTextContent() );
System.out.println("suchChildNodes.item(j).getTextContent() "+suchChildNodes.item(j).getNodeName());
System.out.println("suchChildNodes.item(j).getTextContent() "+suchChildNodes.item(j).getLocalName());
System.out.println("--------------");/
Node node = childNodes.item(i).getAttributes().getNamedItem("rdf:about");
/System.out.println(node.getTextContent());
System.out.println(node.getNodeName());
System.out.println(node.getLocalName());
System.out.println(node.getNodeValue())/;
mapAttributeList.put(suchChildNodes.item(j).getTextContent(), node.getTextContent());
// childNodes.item(i).getAttributes().getNamedItem("rdf:about") -- url status 2 url
// suchChildNodes.item(j).getTextContent() -- name ststus2
// if( (reverseMap.get(tempForDataTypes)).equals( (suchChildNodes.item(j).getTextContent()) ) ) {
/System.out.println("childNodesDataTypesList.item(i).getNodeName() "+childNodes.item(i).getNodeName());
System.out.println(childNodes.item(i).getBaseURI());
p = 1;
// break;
if("rm:AttributeType".equals(childNodesDataTypesList.item(p).getNodeName())){
NodeList suchChildNodesDataTypes = childNodesDataTypesList.item(i).getChildNodes();
for(int k=0; k < suchChildNodesDataTypes.getLength(); k++){
if("dcterms:title".equalsIgnoreCase(suchChildNodesDataTypes.item(j).getNodeName()) ){
System.out.println(" Data Type of "+ mapArtifactNameFromUri.get(tempForDataTypes) +" is : " +suchChildNodesDataTypes.item(j).getTextContent());
}
}
}/
// }
}
}
}
// System.out.println("List of Data Types in this project Area:");
/for (String entryFromListOfDataTypes : list) {
System.out.println("Before: "+entryFromListOfDataTypes);
Pattern pattern = Pattern.compile("[^0-9]");
Matcher matcher = pattern.matcher(entryFromListOfDataTypes);
entryFromListOfDataTypes = entryFromListOfDataTypes.replaceAll("[\+\@\.\^:,]","");
entryFromListOfDataTypes = entryFromListOfDataTypes.replaceAll("[\:,]"," ");
entryFromListOfDataTypes = entryFromListOfDataTypes.replaceAll("[0-9]","");
System.out.println("After: "+entryFromListOfDataTypes);
}/
}
return mapAttributeList;
}
public static String updatingResponse(InputStream tempInputStream, String[] urls, String valueToBeUpdated, String attributedToBeUpdated, int q) throws IOException,
ParserConfigurationException, SAXException, TransformerException {
//urls --> url for artifact to be updated
// value to be update https://hjalmdclm.kpit.com:9443/rm/types/_esUkpkn7EemKYOL47HS_JQ#_esUkqkn7EemKYOL47HS_JQ
InputStream is = tempInputStream;
/InputStreamReader isr = new InputStreamReader(is);
BufferedReader reader = new BufferedReader(isr);
StringBuilder out =new StringBuilder();
String line;
while((line = reader.readLine()) != null) {
out.append(line);
out.append('\n');
}
System.out.println(out.toString());/
String xmlString = null;
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
DocumentBuilder db = dbf.newDocumentBuilder();
Document doc = db.parse(tempInputStream);
DOMSource domSource = new DOMSource(doc);
doc.getDocumentElement().normalize();
// edit the rm:propertytag
/NodeList attribute = doc.getElementsByTagName("rm_property");
Element element = null;/
// one way
// reading the artifact xml for property tag of given attribute status 2
// on this xml properrty tag value will ve rep;aced with the values send in method
if(q==0){
xmlString = null;
NodeList nl1 = doc.getElementsByTagName("rdf:Description");
for(int p=0; p< nl1.getLength(); p++){
NodeList nl2 = nl1.item(p).getChildNodes();
String nameTsr = null;
for(int z=0; z<nl2.getLength(); z++){
if(nl2.item(z).getNodeName().contains("rm_property")){
Node n1 = nl2.item(z);
Element el3 = (Element) n1;
System.out.println(el3.getAttribute("rdf:resource"));
System.out.println(el3.getAttribute(nl2.item(z).getNodeName()));
// URL for enum value . this will be updated to original attribute
nameTsr = el3.getAttribute("rdf:resource");
if(!(el3.getAttribute("rdf:resource").equalsIgnoreCase(valueToBeUpdated))){
continue;
}
else{
// logic to create the tag on which value will be update.
// value after rm:poprty is created here
String attributeToBeUpdatedRefineVariable = mapAttributeList.get(attributedToBeUpdated.trim());
System.out.println("attributeToBeUpdatedRefineVariable : " + attributeToBeUpdatedRefineVariable);
attributeToBeUpdatedRefineVariable = attributeToBeUpdatedRefineVariable.trim();
int index = attributeToBeUpdatedRefineVariable.indexOf("types");
attributeToBeUpdatedRefineVariable = attributeToBeUpdatedRefineVariable.substring(index+6, attributeToBeUpdatedRefineVariable.length());
System.out.println("attributeToBeUpdatedRefineVariable : "+ attributeToBeUpdatedRefineVariable);
NodeList ndl = doc.getElementsByTagName("rdf:Description");
Element ndl2 = (Element) ndl.item(0);
Element ndl21 = (Element) ndl.item(0);
// VALUE TO BE ADDED AS ATTRIBUTE NOT TEXT CONTENT
// ITS SELF CLOSING TAG ATTRIBUTE
Element root = doc.getDocumentElement();
/ Element cd2 = doc.createElement("dcterms:"+attributedToBeUpdated);
cd2.appendChild(doc.createTextNode(valueToBeUpdated));
ndl21.appendChild(cd2);/
Element cd = doc.createElement("rm_property:"+ attributeToBeUpdatedRefineVariable);
cd.setAttribute("rdf:resource", valueToBeUpdated);
//cd.appendChild(doc.createAttribute("rdf:resource="+valueToBeUpdated));
//createAttributeNS(valueToBeUpdated, "rdf:resource"));
//createTextNode(valueToBeUpdated));
ndl2.appendChild(cd);
StringWriter writer = new StringWriter();
StreamResult result = new StreamResult(writer);
TransformerFactory tf = TransformerFactory.newInstance();
Transformer transformer = tf.newTransformer();
transformer.transform(domSource, result);
xmlString=writer.toString();
System.out.println(xmlString);
System.out.println("XML file updated successfully");
return xmlString ;
}
}
}
}
/ for(int i=0; i < childNodesAttributeName.getLength() ; i++){
System.out.println("childNodesAttribute.item(i).getNodeName() : " + childNodesAttributeName.item(i).getNodeName());
if((childNodesAttributeName.item(i).getNodeName()).equalsIgnoreCase("rdf:Description")){
NodeList childOfChildList =childNodesAttributeName.item(i).getChildNodes();
for(int j=0; j< childOfChildList.getLength(); j++){
System.out.println("////// "+childOfChildList.item(j).getNodeName());
System.out.println("childOfChildList.item(j).getTextContent() : "+ childOfChildList.item(j).getTextContent());
// read url and then match it against valueToBeUpdated,
// text content is not correct parameter
Node emp = (Node) childOfChildList.item(j);
System.out.println(childOfChildList.item(j).getNodeName()+"$$$$");
NamedNodeMap nnn2 = emp.getAttributes();
//.getNamedItem(childOfChildList.item(j).getNodeName());
//(childOfChildList.item(j).getNodeName());
Node nnn = nnn2.item(0);
// NodeList nodeListGetPropertyUrl = doc.getElementsByTagName(childOfChildList.item(j).getNodeName());
// String nameOfAttributeToPostValueOn2 = null;
System.out.println(nnn.getTextContent());
System.out.println(nnn.getNodeValue());
System.out.println(nnn.getNodeType());
System.out.println(nnn.getNodeName());
System.out.println(nnn.getLocalName());
System.out.println(nnn.getNamespaceURI());
System.out.println(nnn.getPrefix());
System.out.println(nnn.getBaseURI());
if((childOfChildList.item(j).getNodeName()).contains("rm_property")) {
//&& (nameOfAttributeToPostValueOn2).equalsIgnoreCase(valueToBeUpdated)){
// System.out.println(childOfChildList.item(j).getUserData(""));
String propertyName = childOfChildList.item(j).getNodeName();
// for enum types
// for string Descritpom put if else on tag value null and use other logic
// Using Element logic to read the url value from the tag
Element el = (Element) childOfChildList.item(j);
String nameOfAttributeToPostValueOn = null;
NamedNodeMap nnm = el.getAttributes();
if(el.hasAttribute("rdf:resource")){
el.getAttribute("rdf:resource");
nameOfAttributeToPostValueOn = el+"";
}
// System.out.println("Matching Child Nodes -- "+childNodesAttributeName.item(j).getAttributes().getNamedItem("rdf:resource"));
// Node nodeAttributeName = childOfChildList.item(j).getAttributes().getNamedItem("rdf:resource");
// this uri is the value to be posted on original attribute
// String nameOfAttributeToPostValueOn = nodeAttributeName.getTextContent();
System.out.println("nameOfAttributeToPostValueOn : " + nameOfAttributeToPostValueOn);
//= mapAttributeList.get(attributedToBeUpdated);
String attributeToBeUpdatedRefineVariable = null;
Set<Entry<String, String>> entrySet = mapAttributeList.entrySet();
for (Entry<String, String> entry : entrySet) {
System.out.println(entry.getKey());
if(entry.getKey().equalsIgnoreCase(attributedToBeUpdated.trim())){
attributeToBeUpdatedRefineVariable = entry.getValue();
System.out.println("attributeToBeUpdatedRefineVariable : " + attributeToBeUpdatedRefineVariable);
break;
}
}
int index = attributeToBeUpdatedRefineVariable.indexOf("types");
attributeToBeUpdatedRefineVariable = attributeToBeUpdatedRefineVariable.substring(index+6, attributeToBeUpdatedRefineVariable.length());
System.out.println("attributeToBeUpdatedRefineVariable : "+ attributeToBeUpdatedRefineVariable);
System.out.println("childOfChildList.item(j) : " + childOfChildList.item(j)) ;
NodeList ndl = doc.getElementsByTagName("rdf:Description");
Element ndl2 = (Element) ndl.item(0);
Element root = doc.getDocumentElement();
Element cd = doc.createElement("rm_property:"+ attributeToBeUpdatedRefineVariable);
cd.appendChild(doc.createTextNode(valueToBeUpdated));
ndl2.appendChild(cd);
StringWriter writer = new StringWriter();
StreamResult result = new StreamResult(writer);
TransformerFactory tf = TransformerFactory.newInstance();
Transformer transformer = tf.newTransformer();
transformer.transform(domSource, result);
xmlString=writer.toString();
System.out.println(xmlString);
return xmlString;
}
}
}
}/
return xmlString;
}
else if(q==1){
xmlString = null;
NodeList childNodesAttributeName = doc.getDocumentElement().getChildNodes();
for(int i=0; i < childNodesAttributeName.getLength() ; i++){
System.out.println("childNodesAttribute.item(i).getNodeName() : " + childNodesAttributeName.item(i).getNodeName());
if((childNodesAttributeName.item(i).getNodeName()).equalsIgnoreCase("rdf:Description")){
NodeList childOfChildList =childNodesAttributeName.item(i).getChildNodes();
for(int j=0; j< childOfChildList.getLength(); j++){
if((childOfChildList.item(j).getNodeName()).contains("rm_property")
&& (childOfChildList.item(j).getTextContent()).equalsIgnoreCase(valueToBeUpdated)){
// this is the value to be set in original name , its sent in the method
System.out.println(childOfChildList.item(j).getTextContent());
System.out.println(childOfChildList.item(j).getNodeValue());
System.out.println(childOfChildList.item(j).getNodeName());
String propertyName = childOfChildList.item(j).getNodeName();
mapArtifactNameFromUri.size(); // map for artifacttypes
mapAttributeList.size(); // map for attribute name and urls
String attributeToBeUpdatedRefineVariable = mapAttributeList.get(attributedToBeUpdated);
System.out.println("attributeToBeUpdatedRefineVariable : " + attributeToBeUpdatedRefineVariable);
int index = attributeToBeUpdatedRefineVariable.indexOf("types");
attributeToBeUpdatedRefineVariable = attributeToBeUpdatedRefineVariable.substring(index+6, attributeToBeUpdatedRefineVariable.length());
System.out.println("attributeToBeUpdatedRefineVariable : "+ attributeToBeUpdatedRefineVariable);
System.out.println("childOfChildList.item(j) : " + childOfChildList.item(j)) ;
// set value from description2 onto description
// set description2 value as null also
NodeList ndl = doc.getElementsByTagName("rdf:Description");
Element ndl2 = (Element) ndl.item(0);
Element ndl21 = (Element) ndl.item(0);
Element root = doc.getDocumentElement();
/Element cd2 = doc.createElement("dcterms:"+attributedToBeUpdated);
cd2.appendChild(doc.createTextNode(valueToBeUpdated));
ndl21.appendChild(cd2);/
Element cd = doc.createElement("rm_property:"+ attributeToBeUpdatedRefineVariable);
cd.appendChild(doc.createTextNode(valueToBeUpdated));
ndl2.appendChild(cd);
// removing old node
/Element elmRemove = (Element) childOfChildList.item(j);
elmRemove.removeChild(childOfChildList.item(j));/
StringWriter writer = new StringWriter();
StreamResult result = new StreamResult(writer);
TransformerFactory tf = TransformerFactory.newInstance();
Transformer transformer = tf.newTransformer();
transformer.transform(domSource, result);
xmlString=writer.toString();
System.out.println(xmlString);
/ doc.getDocumentElement().normalize();
TransformerFactory transformerFactory = TransformerFactory.newInstance();
Transformer transformer = transformerFactory.newTransformer();
DOMSource source = new DOMSource(doc);
StreamResult result = new StreamResult(new File("employee_updated.xml"));
transformer.setOutputProperty(OutputKeys.INDENT, "yes");
transformer.transform(source, result);/
System.out.println("XML file updated successfully");
return xmlString ;
}
}
}
}
return xmlString ;
}
// other way check for one to read the property
// create a new tag to u0date a new value in xml with changes attribute value to be updated against the artifact
/if(doc != null)
{
System.out.println(doc.getChildNodes());
NodeList nList = doc.getElementsByTagName("rdf:Description");
for (int i = 0; i < nList.getLength(); i++)
{
Node nNode = nList.item(i);
Element eElement = (Element) nNode;
eElement.appendChild(newElement);
break;
}
} */
// System.out.println("XML IN String format is: \n" + writer.toString());
return xmlString;
}
/
* Create a ist of all Data Types
* Refine the list
* Update Project Area Properties
* @throws URISyntaxException
* @throws OAuthException
* @throws IOException
* @throws RootServicesException
* @throws JazzAuthErrorException
* @throws JazzAuthFailedException
* @throws ResourceNotFoundException
* @throws SAXException
/
public static void readProjectDataTypes(String username, String password, String serverUrl, String projectArea)
throws IOException, OAuthException, URISyntaxException, RootServicesException, JazzAuthFailedException, JazzAuthErrorException, ResourceNotFoundException, SAXException{/
rootServicesHelper = new JazzRootServicesHelper(serverUrl, OSLCConstants.OSLC_RM_V2);
client = rootServicesHelper.initFormClient(username, password);
client.formLogin();
if(client.formLogin()==200){
System.out.println("Logged in");
}
else {
System.out.println("Check the credentials ...");
}
String url = "https://hjalmdclm.kpit.com:9443/rm/types?resourceContext=https://hjalmdclm.kpit.com:9443/rm/process/project-areas/cWM3QMFnEeitSbv70zI1XA";
url = url.trim();
HttpPost connPost = new HttpPost();
HttpGet conn = new HttpGet(url);
conn.setHeader("Content-Type","application/rdf+xml");
conn.setHeader("Accept", "application/rdf+xml");
// conn.setHeader("OSLC-Core-Version", "2.0");
// conn.setHeader("vvc.configuration", "");
// conn.addHeader("Accept-Encoding", "application/x-www-form-urlencoded;charset=UTF-8");
List<String> list = new ArrayList<String>();
HttpResponse httpResponse = client.getHttpClient().execute(conn);
System.out.println(httpResponse.getStatusLine().getStatusCode());
InputStream inputStream = httpResponse.getEntity().getContent();
System.out.println(inputStream);
String line = null;
BufferedReader br = new BufferedReader(new InputStreamReader(inputStream,"UTF8"));
StringBuilder sb = new StringBuilder();
while((line = br.readLine()) != null){
sb.append(line);
}
if(br != null){
br.close();
}
System.out.println(line + sb);
DOMParser parser = new DOMParser();
parser.parse(new InputSource(new StringReader(sb.toString())));
Document document = parser.getDocument();
NodeList childNodes = document.getDocumentElement().getChildNodes();
for(int i=0; i < childNodes.getLength(); i++){
// System.out.println("Child Nodes -- "+childNodes.item(i).getNodeName());
if("rm:AttributeType".equals(childNodes.item(i).getNodeName())){
NodeList suchChildNodes = childNodes.item(i).getChildNodes();
// System.out.println("Matching Child Nodes -- "+childNodes.item(i).getNodeName());
for(int j=0; j < suchChildNodes.getLength(); j++){
if("dcterms:title".equalsIgnoreCase(suchChildNodes.item(j).getNodeName())){
list.add(suchChildNodes.item(j).getTextContent());
System.out.println("Such NOdes --- "+suchildNodes.item(j).getTextContent() );
System.out.println(suchChildNodes.item(j).getLocalName());
System.out.println(suchChilidNodes.item(j).getNodeName());
}
}
}
}
System.out.println("List of Data Types in this project Area:");
for (String entryFromListOfDataTypes : list) {
System.out.println("Before: "+entryFromListOfDataTypes);
Pattern pattern = Pattern.compile("[^0-9]");
Matcher matcher = pattern.matcher(entryFromListOfDataTypes);
entryFromListOfDataTypes = entryFromListOfDataTypes.replaceAll("[\+\@\.\^:,]","");
entryFromListOfDataTypes = entryFromListOfDataTypes.replaceAll("[\:,]"," ");
entryFromListOfDataTypes = entryFromListOfDataTypes.replaceAll("[0-9]","");
System.out.println("After: "+entryFromListOfDataTypes);
}
catalogUrl= rootServicesHelper.getCatalogUrl();
System.out.println(catalogUrl);
serviceProviderUrl = client.lookupServiceProviderUrl(catalogUrl, projectArea);
System.out.println("serviceProviderUrl : "+serviceProviderUrl);
String queryCapabilityUrl= client.lookupQueryCapability(serviceProviderUrl, OSLCConstants.OSLC_RM_V2, OSLCConstants.RM_REQUIREMENT_TYPE);
System.out.println("queryCapabilityUrl : "+queryCapabilityUrl);
String requirementFactory = client.lookupCreationFactory(serviceProviderUrl, OSLCConstants.OSLC_RM_V2, OSLCConstants.RM_REQUIREMENT_TYPE);
System.out.println("requirementFactory 2: "+ requirementFactory);
ITeamRepository repo = TeamPlatform.getTeamRepositoryService().getTeamRepository(rtcURL);
IProcessItemService service = (IProcessItemService) repo.getClientLibrary(IProcessItemService.class);
IProcessDefinition processDefinition = service.findProcessDefinition(processId, IProcessItemService.ALL_PROPERTIES , MONITOR);
ClientResponse datTypeResource = client.getResource("resourceShape.getAbout()", OslcMediaType.APPLICATION_RDF_XML);
// getting Data Type of Instance shape
Map<String, Object> qMapForDataType = new HashMap<>();
qMapForDataType = datTypeResource.getAttributes();
Set<Entry<String, Object>> entrySet = qMapForDataType.entrySet();
System.out.println("");
System.out.println("--------------------------------------");
System.out.println("Printing Instance Shape Q Map ");
for (Entry<String, Object> entry : entrySet) {
System.out.println("Key - "+entry.getKey());
System.out.println("Value - "+entry.getValue());
}
System.out.println("");
System.out.println("----------------------------------------");
/}
/
* Delete duplicate Attributes
/
public static void deleteDuplicateArtifactTypes(Set<String> duplicateArtifactTypesList ){
System.out.println("Deleting Duplicate Attributes now ....");
// access project properties
for (String set : duplicateArtifactTypesList) {
// remove artifact types (duplicateArtifactTypesList)
// persisit
}
}
/
* GetDngArtifactXML
* @return
* @throws Exception
* @throws DOMException
/
public static List<Requirement> getDngArtifactXML(String username, String password, String serverUrl,
String projectArea, String attributeName, String identifier, String projectAreaUUId, String attributeNameToBeChecked) throws DOMException, Exception {
// identifier = "1382";
String xml=null;
Requirement requirement = null;
List<Requirement> listArtifacts = new ArrayList<>();
try{
rootServicesHelper = new JazzRootServicesHelper(serverUrl, OSLCConstants.OSLC_RM_V2);
client = rootServicesHelper.initFormClient(username, password);
client.formLogin();
if(client.formLogin()==200){
System.out.println("Logged in");
}
else {
System.out.println("Check the credentials .");
}
catalogUrl= rootServicesHelper.getCatalogUrl();
System.out.println(catalogUrl);
//throw new RuntimeException("Login Failed"); //8335813657 8225813656
serviceProviderUrl = client.lookupServiceProviderUrl(catalogUrl, projectArea);
System.out.println("serviceProviderUrl : "+serviceProviderUrl);
// System.out.println(rootServicesHelper + " &&& " + client);
String queryCapabilityUrl= client.lookupQueryCapability(serviceProviderUrl, OSLCConstants.OSLC_RM_V2, OSLCConstants.RM_REQUIREMENT_TYPE);
System.out.println("queryCapabilityUrl : "+queryCapabilityUrl);
//serviceProviderUrl,OSLCConstants.OSLC_RM_V2, OSLCConstants.RM_REQUIREMENT_TYPE
// RM_REQUIREMENT_TYPE);
/String requirementFactory = client.lookupCreationFactory(serviceProviderUrl, OSLCConstants.OSLC_RM_V2, OSLCConstants.RM_REQUIREMENT_TYPE);
System.out.println("requirementFactory 2: "+ requirementFactory);/
// Service provider URL maintained as per component or baseline requirements
OslcQueryParameters queryParams = new OslcQueryParameters();
queryParams.setPrefix("dcterms=<http://purl.org/dc/terms/>");
queryParams.setWhere("dcterms:identifier="+ identifier);
queryParams.setSelect("dcterms:title");
OslcQuery query = new OslcQuery(client, queryCapabilityUrl, 10, queryParams);
OslcQueryResult result = query.submit();
String[] urls= result.getMembersUrls();
System.out.println("Number of URLs returned : "+ urls.length);
String artifactTypeUri = null;
String artifactDngId = null;
ClientResponse response=null;
String resourceUri=null;
String typeOfArtifact = null;
// ClientResponse response = client.getResource(resultsUrl[i], OSLCConstants.CT_RDF);
int i=0;
for(String s:urls)
{
requirement = null;
resourceUri=(String)s;
response = client.getResource(resourceUri , OslcMediaType.APPLICATION_RDF_XML);
/ ClientResponse clientResponseAttribute = client.getResource(requirement.getInstanceShape().toString(), OSLCConstants.CT_RDF);
ResourceShape resourceShape = clientResponseAttribute.getEntity(ResourceShape.class);
artifactType = resourceShape.getTitle();/
// for xml element node breakup
xml=response.getEntity(String.class);
System.out.println("Printing XML: "+xml);
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document document = builder.parse(new InputSource(new StringReader(xml)));
NodeList rootElementInstanceShape = document.getElementsByTagName("oslc:instanceShape");
NodeList typeOfArtifactTag = document.getElementsByTagName("rdf:type");
NodeList artifactIdentifier = document.getElementsByTagName("dcterms:identifier");
System.out.println("By Id; : "+document.lookupPrefix("dcterms:identifier"));
System.out.println("artifactIdentifier.length : " +artifactIdentifier.getLength());
for(int j=0; j<rootElementInstanceShape.getLength(); j++){
// tech for self closing tags
Node nNode = rootElementInstanceShape.item(j);
Element eElement = (Element) nNode;
artifactTypeUri = eElement.getAttribute("rdf:resource");
System.out.println("artifact type uri: " + artifactTypeUri );
break;
}
// TO GET TYPE OF ARTIFACT (ARTIFACT OR MODULE)
for(int p=0; p<typeOfArtifactTag.getLength(); p++){
Node nNode = typeOfArtifactTag.item(p);
Element eElement = (Element) nNode;
typeOfArtifact = eElement.getAttribute("rdf:resource");
System.out.println("artifact type name : " + typeOfArtifact );
break;
}
// read non self closing tag tech
for (int k = 0; k<artifactIdentifier.getLength(); k++) {
Node nNode = artifactIdentifier.item(k);
Element eElement = (Element)nNode;
System.out.println("----- "+ eElement.getTextContent());
artifactTypeKMap(artifactTypeUri, eElement.getTextContent(),typeOfArtifact, username,
password, serverUrl, projectArea, projectAreaUUId, attributeNameToBeChecked);
break;
}
/DOMSource domSource = new DOMSource(doc);
doc.getDocumentElement().normalize();/
// for requirement object class methods
/ requirement = response.getEntity(Requirement.class);
if(requirement == null)
{
}
else{
// xml=response.getEntity(String.class);
System.out.println(i++);
System.out.println(requirement);
System.out.println(requirement.getIdentifier());
System.out.println(requirement.getAbout());
System.out.println(requirement.getTitle());
System.out.println(requirement.getRdfTypes());
ResourceShape featureInstanceShape = RmUtil.lookupRequirementsInstanceShapes( serviceProviderUrl, OSLCConstants.OSLC_RM_V2,
OSLCConstants.RM_REQUIREMENT_TYPE, client, artifactType);
ResourceShape resourceShape = clientResponseAttribute.getEntity(ResourceShape.class);
artifactType = resourceShape.getTitle();
ClientResponse clientResponseAttribute = client.getResource(requirement.getInstanceShape().toString(), OSLCConstants.CT_RDF);
ResourceShape resourceShape = clientResponseAttribute.getEntity(ResourceShape.class);
listArtifacts.add(requirement);
}/
break;
}
// artifactTypeKMap(null);
logger.info("Execution completed at " + new Timestamp(System.currentTimeMillis()) );
return listArtifacts;
}
catch(ResourceNotFoundException e){
e.printStackTrace();
//System.out.println(e.getMessage());
}
catch(IOException e){
// System.out.println(e.getMessage());
e.printStackTrace();
}
catch(OAuthException e){
e.printStackTrace();
// System.out.println(e.getMessage());
}
catch(URISyntaxException e){
e.printStackTrace();
//System.out.println(e.getMessage());
}
return listArtifacts;
}
/
* createArtifact
* @return
/
public static boolean createArtifact(String username, String password, String serverUrl, String projectArea,String artifactType, String artifactDescription, String artifactTitle) {
try{
loginToDNG(username, password, serverUrl, projectArea);
String requirementFactory = client.lookupCreationFactory(serviceProviderUrl, OSLCConstants.OSLC_RM_V2, OSLCConstants.RM_REQUIREMENT_TYPE);
Requirement requirement = new Requirement();
ResourceShape featureInstanceShape = RmUtil.lookupRequirementsInstanceShapes( serviceProviderUrl, OSLCConstants.OSLC_RM_V2, OSLCConstants.RM_REQUIREMENT_TYPE, client, artifactType);
requirement .setInstanceShape(featureInstanceShape.getAbout());
requirement.setDescription(artifactDescription);
requirement.setTitle(artifactTitle);
ClientResponse response= client.createResource(requirementFactory, requirement, OslcMediaType.APPLICATION_RDF_XML, OslcMediaType.APPLICATION_RDF_XML);
if (response.getStatusCode() == 200 || response.getStatusCode() == 201) {
return true;
}
}
catch(ResourceNotFoundException e){
System.out.println(e.getMessage());
}
catch(IOException e){
System.out.println(e.getMessage());
}
catch(OAuthException e){
System.out.println(e.getMessage());
}
catch(URISyntaxException e){
System.out.println(e.getMessage());
}
return false;
}
/
DNG to RTC Linking
@return
/
public static boolean dngToRTCLinking(String username, String password, String serverUrl, String projectArea, String excelFilePath){
Map<String, String> mapOut;
boolean result = false;
try {
mapOut = readExcelInputFIle(excelFilePath);
Set<Map.Entry<String, String>> entrySet = mapOut.entrySet();
Iterator<Map.Entry<String, String>> iterator = entrySet.iterator();
while(iterator.hasNext()){
Map.Entry<String, String> keyEntrySet = iterator.next();
String dngId = keyEntrySet.getKey();
String rtcUrl = keyEntrySet.getValue();
result = createRtcLink(username, password, serverUrl, projectArea, dngId, rtcUrl);
return result;
}
}
catch (IOException e) {
e.printStackTrace();
} catch (ResourceNotFoundException e) {
e.printStackTrace();
} catch (RootServicesException e) {
e.printStackTrace();
} catch (JazzAuthFailedException e) {
e.printStackTrace();
} catch (JazzAuthErrorException e) {
e.printStackTrace();
} catch (OAuthException e) {
e.printStackTrace();
} catch (URISyntaxException e) {
e.printStackTrace();
} catch (ParserConfigurationException e) {
e.printStackTrace();
}
return result;
}
/
Create Links to RTC
@return
@throws IOException
@throws OAuthException
@throws URISyntaxException
@throws ResourceNotFoundException
@throws ParserConfigurationException
@throws RootServicesException
@throws JazzAuthFailedException
@throws JazzAuthErrorException
/
public static boolean createRtcLink(String username, String password, String serverUrl, String projectArea, String dngId, String rtcUrl) throws IOException, OAuthException, URISyntaxException, ResourceNotFoundException, ParserConfigurationException, RootServicesException, JazzAuthFailedException, JazzAuthErrorException {
loginToDNG(username, password, serverUrl, projectArea);
OslcQueryParameters queryParams = new OslcQueryParameters();
queryParams.setPrefix("dcterms=<http://purl.org/dc/terms/>");
queryParams.setWhere("dcterms:identifier="+dngId);
queryParams.setSelect("dcterms:title");
OslcQuery query = new OslcQuery(client, queryCapability, queryParams);
OslcQueryResult result = query.submit();
String resultsUrl[] = result.getMembersUrls();
Link[] link = {new Link(new URI(rtcUrl))};
for(int i=0; i <resultsUrl.length; i++) {
ClientResponse response = client.getResource(resultsUrl[i], OSLCConstants.CT_RDF);
Requirement requirement = response.getEntity(Requirement.class);
requirement.setImplementedBy(link);
String primaryText = "Test Primary Text";
Element obj = RmUtil.convertStringToHTML((String)primaryText);
requirement.getExtendedProperties().put(RmConstants.PROPERTY_PRIMARY_TEXT, obj);
ClientResponse updateResponse= client.updateResource(resultsUrl[i], (Object)requirement, OslcMediaType.APPLICATION_RDF_XML, OslcMediaType.APPLICATION_RDF_XML );
//etag);
if (updateResponse.getStatusCode() == 200 || updateResponse.getStatusCode() == 201) {
return true;
}
else {
return false;
}
}
return false;
}
/
Read Input Excel
@return
@throws IOException
/
public static Map<String, String> readExcelInputFIle(String excelFilePath) throws IOException{
FileInputStream inputStream = new FileInputStream(new File(excelFilePath));
Workbook workbook = new XSSFWorkbook(inputStream);
Sheet firstSheet = workbook.getSheetAt(0);
Iterator<Row> iterator = firstSheet.iterator();
String testCaseIdVar;
String requirementIdVar;
DataFormatter df = new DataFormatter();
int c=0;
while (iterator.hasNext()) {
Row nextRow = iterator.next();
Cell cellKey = nextRow.getCell(0);
Cell cellValue = nextRow.getCell(1);
String dngId = df.formatCellValue(cellKey);
String doorsUrl = df.formatCellValue(cellValue);
System.out.println("cellKey "+ dngId);
System.out.println("cellKey "+ doorsUrl);
if(c == 0){
c++;
}
else if (c != 0){
map.put(dngId, doorsUrl);
}
}
return map;
}
/
Main method
*/
public static void main(String[] args) {
System.out.println("Import the Jar in your Project to use it. Thanks ");
}
}
config.properties
serverUrl = https://l-4343:9443/rm
projectArea = BT Testing
attributeName = Requirement
attributeNameToBeChecked = Sta
projectAreaUUId = _EVDqANYaEemj5ODfB-FEPg
#projectAreaUUId = _6WepQEn5EemKYOL47HS_JQ
#url for data types add here
CustomHttpClient.java
package com.kpit.business;
import java.io.IOException;
import java.security.cert.X509Certificate;
import javax.net.ssl.HostnameVerifier;
import javax.net.ssl.HttpsURLConnection;
import javax.net.ssl.SSLException;
import javax.net.ssl.SSLSession;
import javax.net.ssl.SSLSocket;
import org.apache.http.conn.scheme.Scheme;
import org.apache.http.conn.ssl.SSLSocketFactory;
import org.apache.http.impl.client.DefaultHttpClient;
public class CustomHttpClient extends DefaultHttpClient {
public CustomHttpClient() {
super();
SSLSocketFactory socketFactory = SSLSocketFactory.getSocketFactory();
socketFactory.setHostnameVerifier(new CustomHostnameVerifier());
Scheme scheme = (new Scheme("https", socketFactory, 9443));
getConnectionManager().getSchemeRegistry().register(scheme);
}
public class CustomHostnameVerifier implements org.apache.http.conn.ssl.X509HostnameVerifier {
@Override
public boolean verify(String host, SSLSession session) {
HostnameVerifier hv = HttpsURLConnection.getDefaultHostnameVerifier();
return hv.verify(host, session);
}
@Override
public void verify(String host, SSLSocket ssl) throws IOException {
}
@Override
public void verify(String host, X509Certificate cert) throws SSLException {
}
@Override
public void verify(String host, String[] cns, String[] subjectAlts) throws SSLException {
}
}
}
ReadDataTypes.java
package com.kpit.service;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.StringReader;
import java.net.URISyntaxException;
import java.util.ArrayList;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import net.oauth.OAuthException;
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.xerces.parsers.DOMParser;
import org.eclipse.lyo.client.exception.JazzAuthErrorException;
import org.eclipse.lyo.client.exception.JazzAuthFailedException;
import org.eclipse.lyo.client.exception.ResourceNotFoundException;
import org.eclipse.lyo.client.exception.RootServicesException;
import org.eclipse.lyo.client.oslc.OSLCConstants;
import org.eclipse.lyo.client.oslc.jazz.JazzFormAuthClient;
import org.eclipse.lyo.client.oslc.jazz.JazzRootServicesHelper;
import org.w3c.dom.Document;
import org.w3c.dom.NodeList;
import org.xml.sax.InputSource;
import org.xml.sax.SAXException;
public class ReadDataTypes {
private static JazzRootServicesHelper rootServicesHelper = null;
private static JazzFormAuthClient client = null;
private static String catalogUrl = null;
public ReadDataTypes() {
System.out.println("Reading Data Types ");
}
/
* Create a ist of all Data Types
* Refine the list
* Update Project Area Properties
* @throws URISyntaxException
* @throws OAuthException
* @throws IOException
* @throws RootServicesException
* @throws JazzAuthErrorException
* @throws JazzAuthFailedException
* @throws ResourceNotFoundException
* @throws SAXException
/
public static void readProjectDataTypes(String username, String password, String serverUrl, String projectArea)
throws IOException, OAuthException, URISyntaxException, RootServicesException, JazzAuthFailedException, JazzAuthErrorException, ResourceNotFoundException, SAXException{
rootServicesHelper = new JazzRootServicesHelper(serverUrl, OSLCConstants.OSLC_RM_V2);
client = rootServicesHelper.initFormClient(username, password);
client.formLogin();
System.out.println("Reading Data Types ...");
if(client.formLogin()==200){
System.out.println("Logged in");
}
else {
System.out.println("Check the credentials ...");
}
url = url.trim();
// HttpPost connPost = new HttpPost();
HttpGet conn = new HttpGet(url);
conn.setHeader("Content-Type","application/rdf+xml");
conn.setHeader("Accept", "application/rdf+xml");
// conn.setHeader("OSLC-Core-Version", "2.0");
// conn.setHeader("vvc.configuration", "");
// conn.addHeader("Accept-Encoding", "application/x-www-form-urlencoded;charset=UTF-8");
List<String> list = new ArrayList<String>();
HttpResponse httpResponse = client.getHttpClient().execute(conn);
System.out.println(httpResponse.getStatusLine().getStatusCode());
InputStream inputStream = httpResponse.getEntity().getContent();
System.out.println(inputStream);
String line = null;
BufferedReader br = new BufferedReader(new InputStreamReader(inputStream,"UTF8"));
StringBuilder sb = new StringBuilder();
while((line = br.readLine()) != null){
sb.append(line);
}
if(br != null){
br.close();
}
System.out.println(line + sb);
DOMParser parser = new DOMParser();
parser.parse(new InputSource(new StringReader(sb.toString())));
Document document = parser.getDocument();
NodeList childNodes = document.getDocumentElement().getChildNodes();
for(int i=0; i < childNodes.getLength(); i++){
// System.out.println("Child Nodes -- "+childNodes.item(i).getNodeName());
if("rm:AttributeType".equals(childNodes.item(i).getNodeName())){
NodeList suchChildNodes = childNodes.item(i).getChildNodes();
// System.out.println("Matching Child Nodes -- "+childNodes.item(i).getNodeName());
for(int j=0; j < suchChildNodes.getLength(); j++){
if("dcterms:title".equalsIgnoreCase(suchChildNodes.item(j).getNodeName())){
list.add(suchChildNodes.item(j).getTextContent());
/System.out.println("Such Child NOdes --- "+suchChildNodes.item(j).getTextContent() );
System.out.println(suchChildNodes.item(j).getLocalName());
System.out.println(suchChildNodes.item(j).getNodeName())/;
}
}
}
}
System.out.println("List of Data Types in this project Area:");
for (String entryFromListOfDataTypes : list) {
System.out.println("Before: "+entryFromListOfDataTypes);
Pattern pattern = Pattern.compile("[^0-9]");
Matcher matcher = pattern.matcher(entryFromListOfDataTypes);
entryFromListOfDataTypes = entryFromListOfDataTypes.replaceAll("[\+\@\.\^:,]","");
entryFromListOfDataTypes = entryFromListOfDataTypes.replaceAll("[\_:,]"," ");
entryFromListOfDataTypes = entryFromListOfDataTypes.replaceAll("[0-9]","");
System.out.println("After: "+entryFromListOfDataTypes);
}
/ catalogUrl= rootServicesHelper.getCatalogUrl();
System.out.println(catalogUrl);
serviceProviderUrl = client.lookupServiceProviderUrl(catalogUrl, projectArea);
System.out.println("serviceProviderUrl : "+serviceProviderUrl);
String queryCapabilityUrl= client.lookupQueryCapability(serviceProviderUrl, OSLCConstants.OSLC_RM_V2, OSLCConstants.RM_REQUIREMENT_TYPE);
System.out.println("queryCapabilityUrl : "+queryCapabilityUrl);
String requirementFactory = client.lookupCreationFactory(serviceProviderUrl, OSLCConstants.OSLC_RM_V2, OSLCConstants.RM_REQUIREMENT_TYPE);
System.out.println("requirementFactory 2: "+ requirementFactory);
/
/ ITeamRepository repo = TeamPlatform.getTeamRepositoryService().getTeamRepository(rtcURL);
IProcessItemService service = (IProcessItemService) repo.getClientLibrary(IProcessItemService.class);
IProcessDefinition processDefinition = service.findProcessDefinition(processId, IProcessItemService.ALL_PROPERTIES , MONITOR);
/
/
ClientResponse datTypeResource = client.getResource("resourceShape.getAbout()", OslcMediaType.APPLICATION_RDF_XML);
// getting Data Type of Instance shape
Map<String, Object> qMapForDataType = new HashMap<>();
qMapForDataType = datTypeResource.getAttributes();
Set<Entry<String, Object>> entrySet = qMapForDataType.entrySet();
System.out.println("");
System.out.println("--------------------------------------");
System.out.println("Printing Instance Shape Q Map ");
for (Entry<String, Object> entry : entrySet) {
System.out.println("Key - "+entry.getKey());
System.out.println("Value - "+entry.getValue());
}
System.out.println("");
System.out.println("----------------------------------------");*/
}
}
Log4j.xml
<!-- <?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE log4j:configuration SYSTEM "log4j.dtd">
<log4j:configuration>
<appender name="FILE" class="org.apache.log4j.FileAppender">
<param name="file" value="C:\Users\vaibhavs18\workspace\CleanUp Script\log.txt"/>
<param name="immediateFlush" value="true"/>
<param name="threshold" value="debug"/>
<param name="append" value="false"/>
<layout class="org.apache.log4j.PatternLayout">
<param name="conversionPattern" value="%m%n"/>
</layout>
</appender>
<logger name="log4j.rootLogger" additivity="false">
<level value="DEBUG"/>
<appender-ref ref="FILE"/>
</logger>
<logger name="log4j.rootLogger" additivity="false">
<level value="INFO"/>
<appender-ref ref="FILE"/>
</logger>
</log4j:configuration> -->
log4l.properties
# Root logger option
log4j.rootLogger=INFO, file
# configuration to print into file
log4j.appender.file=org.apache.log4j.RollingFileAppender
log4j.appender.file.File=D:\log\logging.log
log4j.appender.file.MaxFileSize=12MB
log4j.appender.file.MaxBackupIndex=10
log4j.appender.file.layout=org.apache.log4j.PatternLayout
log4j.appender.file.layout.ConversionPattern=%d{yyyy-MM-dd HH:mm:ss} %-5p %c{1}:%L - %m%n