How to create Cross component link in a GC enabled PA programmatically
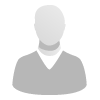
One answer
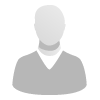
Hi,
I found the code to do it. Hope it helps
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Properties;
import java.util.Set;
import javax.xml.namespace.QName;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import org.apache.wink.client.ClientResponse;
import org.eclipse.lyo.client.exception.JazzAuthErrorException;
import org.eclipse.lyo.client.exception.JazzAuthFailedException;
import org.eclipse.lyo.client.exception.ResourceNotFoundException;
import org.eclipse.lyo.client.exception.RootServicesException;
import org.eclipse.lyo.client.oslc.OSLCConstants;
import org.eclipse.lyo.client.oslc.jazz.JazzFormAuthClient;
import org.eclipse.lyo.client.oslc.jazz.JazzRootServicesHelper;
import org.eclipse.lyo.client.oslc.resources.OslcQuery;
import org.eclipse.lyo.client.oslc.resources.OslcQueryParameters;
import org.eclipse.lyo.client.oslc.resources.Requirement;
import org.eclipse.lyo.oslc4j.core.model.OslcMediaType;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import net.oauth.OAuthException;
public class GetUriFromIdentifier {
public static void main(String[] args) throws Exception{
// TODO Auto-generated method stub
FileInputStream fileInputStream= new FileInputStream(new File("./configuration.properties"));
Properties properties= new Properties();
properties.load(fileInputStream);
String serverUrl= properties.getProperty("repositoryUrl");
String userName= properties.getProperty("username");
String password= properties.getProperty("password");
String projectAreaName= properties.getProperty("paName");
String gcStreamID= properties.getProperty("gcStreamID");
String src_artifact_Id="6259";
String target_artifact_Ids="678340, 689720";
String[] target_Ids=target_artifact_Ids.split(",");
GetUriFromIdentifier object = new GetUriFromIdentifier();
JazzRootServicesHelper helper = new JazzRootServicesHelper(serverUrl, OSLCConstants.OSLC_RM_V2);
JazzFormAuthClient client= helper.initFormClient(userName, password, serverUrl);
if(client.formLogin()==200){
System.out.println("Log-in SUCCESS!");
}else{
//System.out.println("Log-in status:"+client.formLogin());
}
String catalogUrl= helper.getCatalogUrl();
//System.out.println("Catalog Url:\n"+catalogUrl);
String serviceProviderUrl1=client.lookupServiceProviderUrl(catalogUrl, projectAreaName);
//System.out.println("Service provider url:\n"+serviceProviderUrl1);
String queryCapabilityUrl= client.lookupQueryCapability(serviceProviderUrl1, OSLCConstants.OSLC_RM_V2, OSLCConstants.RM_REQUIREMENT_TYPE);
//System.out.println("Query capability url:\n"+queryCapabilityUrl);
String requirementFactory = client.lookupCreationFactory(serviceProviderUrl1, OSLCConstants.OSLC_RM_V2, OSLCConstants.RM_REQUIREMENT_TYPE);
//System.out.println("RF:\n"+requirementFactory);
String src_arti_uri= object.getArtifactURIs(userName, password, serverUrl, projectAreaName, src_artifact_Id, client, queryCapabilityUrl, gcStreamID);
String[] links= new String[target_Ids.length];
for(int i=0;i<target_Ids.length;i++){
links[i]=object.getArtifactURIs(userName, password, serverUrl, projectAreaName, target_Ids[i].trim(), client, queryCapabilityUrl, gcStreamID);
}
ClientResponse response = client.getResource(src_arti_uri , OslcMediaType.APPLICATION_RDF_XML);
String etag = response.getHeaders().getFirst(OSLCConstants.ETAG);
Requirement requirement= response.getEntity(Requirement.class);
Map<QName, Object> getMap= requirement.getExtendedProperties();
String namespaceURI="http://bosch.com/dng/link-type/";
String localPart="Satisfaction";
String prefix="j.0";
QName defaultQName= new QName(namespaceURI, localPart, prefix);
ArrayList<URI> satisfiesLinksList=new ArrayList<URI>();
URI oneLink;
ArrayList<String> arrayList= new ArrayList<>();
if(getMap.containsKey(defaultQName)){
if(getMap.get(defaultQName) instanceof URI){
oneLink=(URI)getMap.get(defaultQName);
satisfiesLinksList.add(oneLink);
}else{
satisfiesLinksList=(ArrayList<URI>)getMap.get(defaultQName);
}
}
for(String link: links){
satisfiesLinksList.add(new URI(link));
}
getMap.put(defaultQName, satisfiesLinksList);
requirement.setExtendedProperties(getMap);
ClientResponse updateResponse= client.updateResource(src_arti_uri , (Object)requirement, OslcMediaType.APPLICATION_RDF_XML, OslcMediaType.APPLICATION_RDF_XML, etag);
System.out.println("Status: "+updateResponse.getStatusCode());
}
public String getArtifactURIs(String username, String password, String serverURL, String projectAreaName,
String artifactId, JazzFormAuthClient client, String queryCapabilityUrl, String stream) throws Exception {
// TODO Auto-generated method stub
OslcQueryParameters queryParams = new OslcQueryParameters();
queryParams.setPrefix("dcterms=<http://purl.org/dc/terms/>");
queryParams.setWhere("dcterms:identifier="+artifactId);
//queryParams.setSelect("dcterms:title");
OslcQuery query = new OslcQuery(client, queryCapabilityUrl, 10, queryParams);
Map<String, String> headers = new HashMap<>();
headers.put("oslc.configuration",stream);
String url=query.getQueryUrl();
ClientResponse response = client.getResource(url, headers);
InputStream inputStream = response.getEntity(InputStream.class);
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
DocumentBuilder db = dbf.newDocumentBuilder();
Document doc = db.parse(inputStream);
doc.getDocumentElement().normalize();
String[] uris = null;
if(doc != null)
{
NodeList nList = doc.getElementsByTagName("oslc_rm:Requirement");
if(nList.getLength()==0){
nList = doc.getElementsByTagName("oslc_rm:RequirementCollection");
}
uris=new String[nList.getLength()];
for (int i = 0; i < nList.getLength(); i++)
{
Node nNode = nList.item(i);
Element eElement = (Element) nNode;
String uri=eElement.getAttribute("rdf:about");
uris[i]=uri;
}
}
return uris[0];
}
}