How to programmatically add components to workspace

IScmService scmService = ... IContributorHandle contributorH = ... IWorkspaceHandle stream = ... IWorkspace wks = scmService.createWorkspace(contributorH, wksName, wksDescription, stream, null, stream, null, null, IRepositoryProgressMonitor.ITEM_FACTORY.createItem(monitor)).getWorkspace();
IComponentHandle componentH = ...
IWorkspaceHandle targetH = ...
scmService.addComponentFromWorkspace(wks, componentH, targetH, false, IScmService.DELTA_PER_INVOCATION, null, IRepositoryProgressMonitor.ITEM_FACTORY.createItem(monitor));
4 answers

// Add new components Collectioncomponents = extComponents.values(); for (Object comp : components) { IComponentHandle cHandle = (IComponentHandle) comp; workspaceConnection.applyComponentOperations(Collections .singletonList(workspaceConnection.componentOpFactory() .addComponent(cHandle, false)), true, monitor); }
Comments
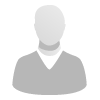
The snippet is the correct way to do it. Using the service is a different layer, not recommended, and is also the deprecated call.

Tim, I think the snippets should be reworked, there are several deprecated methods in usage and I actually had to dig into the deprecated call to figure out the ones not deprecated.
1 vote

We are using the IScmService service beacuse we are on a server extension, not on a client...
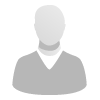
IScmService#updateComponents2(...) is the current call to add a component to a workspace.

IWorkspace wks = ...
List<FlowEntry> flows = ((Workspace) wks).getFlows();
List<FlowEntry> acceptFlows = new ArrayList<FlowEntry>();
List<FlowEntry> deliverFlows = new ArrayList<FlowEntry>();
for (FlowEntry flow : flows) {
if ((flow.getFlags() & FlowFlags.ACCEPT ) != 0) {
acceptFlows.add((FlowEntry) EcoreUtil.copy((EObject) flow));
} else if ((flow.getFlags() & FlowFlags.DELIVER) != 0) {
deliverFlows.add((FlowEntry) EcoreUtil.copy((EObject) flow));
}
}
FlowEntry flow = ScmFactory.eINSTANCE.createFlowEntry();
flow.setTargetWorkspace(target);
flow.setFlags(FlowFlags.DELIVER);
deliverFlows.add(flow);
CurrentFlows currentFlows = (CurrentFlows) EcoreUtil.copy((EObject) ((Workspace) wks).getCurrentFlows());
IComponentHandle[] components = ...
CurrentFlows[] currentComponentFlows = ...
scmService.setWorkspaceFlows(wks, acceptFlows.toArray(new FlowEntry[0]), deliverFlows.toArray(new FlowEntry[0]), currentFlows, components, currentComponentFlows, null, IRepositoryProgressMonitor.ITEM_FACTORY.createItem(monitor));

IWorkspace wks = scmService.createWorkspace(contributorH, wksName, wksDescription, stream, null, stream, null, null, IRepositoryProgressMonitor.ITEM_FACTORY.createItem(monitor)).getWorkspace();
IComponentHandle componentH = ...
IWorkspaceHandle seedH = ...
IComponentOpDescriptor op = IComponentOpDescriptor.FACTORY.addComponent(componentH, seedH, false);
scmService.updateComponents(wksH, null, new IComponentOpDescriptor[]{ op }, false, IScmService.DELTA_PER_INVOCATION, null, IRepositoryProgressMonitor.ITEM_FACTORY.createItem(monitor));
Comments
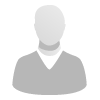
I don't know what you mean by nothing changed. Can you post what you did to check if the component was added to the workspace?

We mean "nothing changed despite to use a IComponentOpDescriptor operation".
We run the code above by a save work-item post-operation, we searched for workspace created in to repository, then we opened it.

Comments
SEC Servizi
Sep 09 '13, 10:33 a.m.During further investigation, it appears that added components are dropped by the save transaction. Any advice?