Authentication Issue to RTC server (v6.0.2) using c#

Hi,
5 answers

Your code is not that different from that in the blog. You need to understand what your code does and how to debug it. Regardless the programming/scripting language of choice, the process of authentication is very simple.
1. Try to access a protected resource, to verify whether you're already logged in, and get the JSESSIONID at the same time.
2. If not yet logged in, POST to /<app>/authenticated/j_security_check with j_username and j_password for authentication, where <app> can be "jts", "ccm", "qm", "jazz" or whatever the root context of your Jazz application is assigned to.
Depending on what value you pass on to the variable "_rtcServerURL", you may not have the correct URL to POST at all. Please double check.
Comments
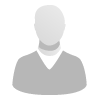
You don't need to add any extra headers for the POST request, but make sure you have retained all the cookies and used them with this request. Other than that, I can imagine what can go wrong - as I said, the authentication process is very simple.

After the step 1 call, in the response header there is a "Set-Cookie" header. Is that what you referring? Do I need to put that in header when I POST in step 2? If yes, how to do that?
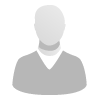
You should retain all the cookies. I'm not familiar with C# and you probably need to ask such question on Microsoft's developer forum.

This is what I added to pass the cookie, still got "authfailed":

Thanks Don!
Comments
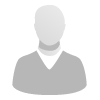
One pitfall that you should watch out for and that you might want to check is if your password uses any characters that need to be HTML encoded. The first time I developed an API application, it worked for me and then I gave it to someone else to use and it locked their account. It worked for me because my password didn't have any unusual characters, but theirs did. My code wasn't HTML encoding the password so I was authenticating fine, but they were not.
1 vote

I don't know C# but here is the java code I use:
HttpPost formPost = new HttpPost(jtsURI + "/j_security_check");
List<NameValuePair> nvps = new ArrayList<NameValuePair>();
nvps.add(new BasicNameValuePair("j_username", login));
nvps.add(new BasicNameValuePair("j_password", password));
formPost.setEntity(new UrlEncodedFormEntity(nvps, HTTP.UTF_8));
HttpResponse formResponse = httpClient.execute(formPost);
Note that I am going to JTS and not RTC and as previously mentioned, the username and password are encoded (which you might be doing with Encoding.UTF8.GetBytes, but I am not familiar with C# libraries). I don't add any headers.

This line looks wrong to me:
String _authString = "j_username=" + _userName + "&j_password=" + _password; //create authentication string
You don't encode the '&' separator character in form-encoded input. Encoding it makes it part of the value of the "j_username" parameter, instead of indicating the end of the value of that parameter and the start of another one. Try using
String _authString = "j_username=" + _userName + "&j_password=" + _password; //create authentication string
You might find https://jazz.net/wiki/bin/view/Main/NativeClientAuthentication interesting - it describes how to deal with all the authenticaiton protocols that Jazz servers can be configured with.

I was able to use the sample solution from this zip file to successfully access project area on our server.
Comments
Ulf Arne Bister
Jul 25 '17, 5:47 p.m.Is the sample code mentioned here working better for you?
http://blog.boriskuschel.com/2012/02/c-with-visual-studio-and-rational-team.html