Programaticaly How to get list of Work Items for a specific time period?
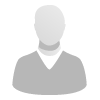
Accepted answer
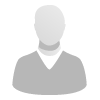
Probably, the most easy way is to use Reports restAPI.
https://jazz.net/wiki/bin/view/Main/ReportsRESTAPI#Resources_provided_by_RTC
For example, in you case:
https://<your-server>:9443/ccm/rpt/repository/workitem?fields=workitem/workItem[creationDate > 2014-4-14T10:10:53.000-0900 and creationDate < 2014-4-18T10:10:53.000-0900](id|summary)
https://jazz.net/wiki/bin/view/Main/ReportsRESTAPI#Resources_provided_by_RTC
For example, in you case:
https://<your-server>:9443/ccm/rpt/repository/workitem?fields=workitem/workItem[creationDate > 2014-4-14T10:10:53.000-0900 and creationDate < 2014-4-18T10:10:53.000-0900](id|summary)
One other answer
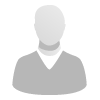
You can also do it using the Java APIs although you have to build the SQL yourself. For example:
Term itemsToQuery = new Term(Operator.AND); //AND all my things together
IQueryableAttributeFactory factory = QueryableAttributes.getFactory(IWorkItem.ITEM_TYPE);
IQueryableAttribute createDateAttribute = factory.findAttribute(myProjectArea, (IWorkItem.CREATION_DATE_PROPERTY), auditableClient, monitor);
Timestamp t = new Timestamp(2014 - 1900, 3, 22, 23, 59, 0, 0); //4/22/2014 00:00 - 4/22/2014 23:59
AttributeExpression startDateExpression = new AttributeExpression(creationDateAttribute,
AttributeOperation.AFTER,
t);
itemsToQuery.add(startDateExpression);
Then do the same thing with another Timestamp for the date you want and do a endDateExpress with the same creationDateAttribute, AttributeOperation.BEFORE, and the other Timestamp.
Then create a statement:
Statement s = new Statement(new SelectClause(), itemsToQuery, new SortCriteria[] { sortByIdSortCriteria }); //sortByIdSortCriteria is a SortCriteria object
And then the query uses the queryClient:
IQueryResult<IResolvedResult<IWorkItem>> results = null;
results = queryClient.getResolvedExpressionResults(myProjectArea, s, IWorkItem.FULL_PROFILE);
Then you can do results.next().getItem() to get the matchingWorkItems.
Timestamp tt = new Timestamp(2014 - 1900, 3, 28, 0, 0, 0, 0); //4/22/2014 00:00 - 4/22/2014 23:59
AttributeExpression endDateExpression = new AttributeExpression(creationDateAttribute,
AttributeOperation.BEFORE,
tt);
newItemsToBridgeToJava.add(startDateExpression);
/* Sort by id */
SortCriteria byId = new SortCriteria(wasRepo.findQueryableAttribute(IWorkItem.ID_PROPERTY), true);
/* Create statement */
Statement s = new Statement(new SelectClause(), newItemsToBridgeToJava, new SortCriteria[] { byId });
Term itemsToQuery = new Term(Operator.AND); //AND all my things together
IQueryableAttributeFactory factory = QueryableAttributes.getFactory(IWorkItem.ITEM_TYPE);
IQueryableAttribute createDateAttribute = factory.findAttribute(myProjectArea, (IWorkItem.CREATION_DATE_PROPERTY), auditableClient, monitor);
Timestamp t = new Timestamp(2014 - 1900, 3, 22, 23, 59, 0, 0); //4/22/2014 00:00 - 4/22/2014 23:59
AttributeExpression startDateExpression = new AttributeExpression(creationDateAttribute,
AttributeOperation.AFTER,
t);
itemsToQuery.add(startDateExpression);
Then do the same thing with another Timestamp for the date you want and do a endDateExpress with the same creationDateAttribute, AttributeOperation.BEFORE, and the other Timestamp.
Then create a statement:
Statement s = new Statement(new SelectClause(), itemsToQuery, new SortCriteria[] { sortByIdSortCriteria }); //sortByIdSortCriteria is a SortCriteria object
And then the query uses the queryClient:
IQueryResult<IResolvedResult<IWorkItem>> results = null;
results = queryClient.getResolvedExpressionResults(myProjectArea, s, IWorkItem.FULL_PROFILE);
Then you can do results.next().getItem() to get the matchingWorkItems.
Timestamp tt = new Timestamp(2014 - 1900, 3, 28, 0, 0, 0, 0); //4/22/2014 00:00 - 4/22/2014 23:59
AttributeExpression endDateExpression = new AttributeExpression(creationDateAttribute,
AttributeOperation.BEFORE,
tt);
newItemsToBridgeToJava.add(startDateExpression);
/* Sort by id */
SortCriteria byId = new SortCriteria(wasRepo.findQueryableAttribute(IWorkItem.ID_PROPERTY), true);
/* Create statement */
Statement s = new Statement(new SelectClause(), newItemsToBridgeToJava, new SortCriteria[] { byId });