Need assistance with RTC Query Java Code
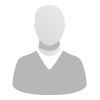
Hopefully Ralph Schoon will see this. I used the code he provided from his blog work items for automation, but receive a few compilation errors and I was wondering if I could get help resolving those.
public class QueryRTCRunSave {
public static void main(String args[]) {
//creates a progress monitor
IProgressMonitor monitor = new NullProgressMonitor();
//stores login credentials in strings
String repositoryURI = "https://rtc.domain.com/jazz";
String userId = "myid";
String password = "****";
//client initializes itself
TeamPlatform.startup();
try {
ITeamRepository repo = logIntoTeamRepository(repositoryURI,
userId, password, monitor);
repo.logout();
} catch (TeamRepositoryException e) {
//this will handle any repository exceptions such as login problems
e.printStackTrace();
//this shuts down the platform
} finally {
TeamPlatform.shutdown();
}
}
private static ITeamRepository logIntoTeamRepository(String repositoryURI,
String userId, String password, IProgressMonitor monitor)
throws TeamRepositoryException {
System.out.println("Trying to log into repository: " + repositoryURI);
TeamPlatform.startup();
ITeamRepository teamRepository = TeamPlatform
.getTeamRepositoryService().getTeamRepository(repositoryURI);
teamRepository.registerLoginHandler(new LoginHandler(userId, password));
teamRepository.login(monitor);
System.out.println("Login succeeded.");
return teamRepository;
}
private static class LoginHandler implements ILoginHandler, ILoginInfo {
private String fUserId;
private String fPassword;
private LoginHandler(String userId, String password) {
fUserId = userId;
fPassword = password;
}
public String getUserId() {
return fUserId;
}
public String getPassword() {
return fPassword;
}
public ILoginInfo challenge(ITeamRepository repository) {
return this;
}
}
/* Do all of my work with RTC servers here. */
//calls the method findpersonalquery
IQueryDescriptor query = findPersonalQuery(projectArea, "All Work Items", monitor);<-- projectArea cannot be resolved to a variable
//method that Finds personal queries stored in repository by project area,name
public static IQueryDescriptor findPersonalQuery(IProjectArea projectArea,
String queryName, IProgressMonitor monitor)
throws TeamRepositoryException {
// Get the required client libraries
ITeamRepository teamRepository = (ITeamRepository)projectArea.getOrigin();
IWorkItemClient workItemClient = (IWorkItemClient) teamRepository.getClientLibrary(
IWorkItemClient.class);
IQueryClient queryClient = workItemClient.getQueryClient();
// Get the current user.
IContributor loggedIn = teamRepository.loggedInContributor();
IQueryDescriptor queryToRun = null;
// Get all queries of the user in this project area.
List queries = queryClient.findPersonalQueries(
projectArea.getProjectArea(), loggedIn,
QueryTypes.WORK_ITEM_QUERY,
IQueryDescriptor.FULL_PROFILE, monitor);
// Find a query with a matching name
for (Iterator iterator = queries.iterator(); iterator.hasNext();) {
IQueryDescriptor iQueryDescriptor = (IQueryDescriptor) iterator.next();
if (iQueryDescriptor.getName().equals(queryName)) {
queryToRun = iQueryDescriptor;
break;
}
}
return queryToRun;
}
//to get unresolved query results stored, you pass query as a parameter
IQueryResult unresolvedResults = queryClient.getQueryResults(queryToRun);<-- queryClient cannot be resolved, queryToRun cannot be resolved to a variable
//calls the method processUnresolvedResults
processUnresolvedResults(projectArea, unresolvedResults, loadProfile, monitor);<-- return type for this method is missing
//method that processes the unresolvedResults and stores in file
public static void processUnresolvedResults(IProjectArea projectArea , IQueryResult results,
ItemProfile profile, IProgressMonitor monitor)
throws TeamRepositoryException {
// Get the required client libraries
ITeamRepository teamRepository = (ITeamRepository)projectArea.getOrigin();
IWorkItemClient workItemClient = (IWorkItemClient)teamRepository.getClientLibrary(IWorkItemClient.class);
IAuditableCommon auditableCommon = (IAuditableCommon)teamRepository.getClientLibrary(IAuditableCommon.class);
long processed = 0;
while (results.hasNext(monitor)) {<--has next method in IQueryResult is not applicable for arguments IProgressMonitor
IResult result = (IResult) results.next(monitor);<--next(IProgressMonitor)is undefined for type IQueryResult
IWorkItem workItem = auditableCommon.resolveAuditable(
(IAuditableHandle) result.getItem(), profile, monitor);
// Do something with the work item here(save to csv or xml)
processed++;
}
System.out.println("Processed results: " + processed);
}
}
public class QueryRTCRunSave {
public static void main(String args[]) {
//creates a progress monitor
IProgressMonitor monitor = new NullProgressMonitor();
//stores login credentials in strings
String repositoryURI = "https://rtc.domain.com/jazz";
String userId = "myid";
String password = "****";
//client initializes itself
TeamPlatform.startup();
try {
ITeamRepository repo = logIntoTeamRepository(repositoryURI,
userId, password, monitor);
repo.logout();
} catch (TeamRepositoryException e) {
//this will handle any repository exceptions such as login problems
e.printStackTrace();
//this shuts down the platform
} finally {
TeamPlatform.shutdown();
}
}
private static ITeamRepository logIntoTeamRepository(String repositoryURI,
String userId, String password, IProgressMonitor monitor)
throws TeamRepositoryException {
System.out.println("Trying to log into repository: " + repositoryURI);
TeamPlatform.startup();
ITeamRepository teamRepository = TeamPlatform
.getTeamRepositoryService().getTeamRepository(repositoryURI);
teamRepository.registerLoginHandler(new LoginHandler(userId, password));
teamRepository.login(monitor);
System.out.println("Login succeeded.");
return teamRepository;
}
private static class LoginHandler implements ILoginHandler, ILoginInfo {
private String fUserId;
private String fPassword;
private LoginHandler(String userId, String password) {
fUserId = userId;
fPassword = password;
}
public String getUserId() {
return fUserId;
}
public String getPassword() {
return fPassword;
}
public ILoginInfo challenge(ITeamRepository repository) {
return this;
}
}
/* Do all of my work with RTC servers here. */
//calls the method findpersonalquery
IQueryDescriptor query = findPersonalQuery(projectArea, "All Work Items", monitor);<-- projectArea cannot be resolved to a variable
//method that Finds personal queries stored in repository by project area,name
public static IQueryDescriptor findPersonalQuery(IProjectArea projectArea,
String queryName, IProgressMonitor monitor)
throws TeamRepositoryException {
// Get the required client libraries
ITeamRepository teamRepository = (ITeamRepository)projectArea.getOrigin();
IWorkItemClient workItemClient = (IWorkItemClient) teamRepository.getClientLibrary(
IWorkItemClient.class);
IQueryClient queryClient = workItemClient.getQueryClient();
// Get the current user.
IContributor loggedIn = teamRepository.loggedInContributor();
IQueryDescriptor queryToRun = null;
// Get all queries of the user in this project area.
List queries = queryClient.findPersonalQueries(
projectArea.getProjectArea(), loggedIn,
QueryTypes.WORK_ITEM_QUERY,
IQueryDescriptor.FULL_PROFILE, monitor);
// Find a query with a matching name
for (Iterator iterator = queries.iterator(); iterator.hasNext();) {
IQueryDescriptor iQueryDescriptor = (IQueryDescriptor) iterator.next();
if (iQueryDescriptor.getName().equals(queryName)) {
queryToRun = iQueryDescriptor;
break;
}
}
return queryToRun;
}
//to get unresolved query results stored, you pass query as a parameter
IQueryResult unresolvedResults = queryClient.getQueryResults(queryToRun);<-- queryClient cannot be resolved, queryToRun cannot be resolved to a variable
//calls the method processUnresolvedResults
processUnresolvedResults(projectArea, unresolvedResults, loadProfile, monitor);<-- return type for this method is missing
//method that processes the unresolvedResults and stores in file
public static void processUnresolvedResults(IProjectArea projectArea , IQueryResult results,
ItemProfile profile, IProgressMonitor monitor)
throws TeamRepositoryException {
// Get the required client libraries
ITeamRepository teamRepository = (ITeamRepository)projectArea.getOrigin();
IWorkItemClient workItemClient = (IWorkItemClient)teamRepository.getClientLibrary(IWorkItemClient.class);
IAuditableCommon auditableCommon = (IAuditableCommon)teamRepository.getClientLibrary(IAuditableCommon.class);
long processed = 0;
while (results.hasNext(monitor)) {<--has next method in IQueryResult is not applicable for arguments IProgressMonitor
IResult result = (IResult) results.next(monitor);<--next(IProgressMonitor)is undefined for type IQueryResult
IWorkItem workItem = auditableCommon.resolveAuditable(
(IAuditableHandle) result.getItem(), profile, monitor);
// Do something with the work item here(save to csv or xml)
processed++;
}
System.out.println("Processed results: " + processed);
}
}
3 answers
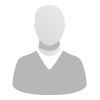
and another answer built from Ralph's example
a full workitem example here
https://jazz.net/forum/questions/94776/assertionfailedexception-problem-with-getting-the-values-of-attributes
second post.
watch out for the forum adding extra double quotes down near the bottom and lowercasing the datatype names.
this will print out all the attributes of all the workitems returned in the query. takes 5 parms
repo, userid, password, projectname, queryname
a full workitem example here
https://jazz.net/forum/questions/94776/assertionfailedexception-problem-with-getting-the-values-of-attributes
second post.
watch out for the forum adding extra double quotes down near the bottom and lowercasing the datatype names.
this will print out all the attributes of all the workitems returned in the query. takes 5 parms
repo, userid, password, projectname, queryname
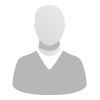
You must initialize projectArea
I've attached similar method which find shared query
IProjectArea projectArea = null;
ITeamRepository teamRepository = null;
String sProjectAreaName="your Project Area";
IProcessClientService processClient = (IProcessClientService) teamRepository.getClientLibrary( IProcessClientService.class);
URI uri = URI.create(sProjectAreaName.replaceAll(" ","%20"));
projectArea = (IProjectArea) processClient.findProcessArea(uri,null,null);
List<IProjectArea> sharingTargets =new ArrayList<IProjectArea>();
sharingTargets.add(projectArea);
IQueryDescriptor sharedQuery = findSharedQuery(projectArea,sharingTargets,sQueryName,monitor);
IQueryDescriptor findSharedQuery(IProjectArea projectArea, List<IProjectArea> sharingTargets, String queryName,IProgressMonitor monitor) throws TeamRepositoryException
{
ITeamRepository teamRepository =(ITeamRepository) projectArea.getOrigin();
IWorkItemClient workItemClient =(IWorkItemClient) teamRepository.getClientLibrary(IWorkItemClient.class);
IQueryClient queryClient = workItemClient.getQueryClient();
IQueryDescriptor queryToRun = null;
List<IQueryDescriptor> queries = queryClient.findSharedQueries(
projectArea.getProjectArea(), sharingTargets,
QueryTypes.WORK_ITEM_QUERY, IQueryDescriptor.FULL_PROFILE,
monitor);
for (Iterator<IQueryDescriptor> iterator = queries.iterator();
iterator.hasNext();) {
IQueryDescriptor iQueryDescriptor =
(IQueryDescriptor) iterator.next();
if (iQueryDescriptor.getName().equals(queryName)) {
queryToRun = iQueryDescriptor;
break;
}
}
if(queryToRun == null) {
logger.error("Unable to find the shared query - " + queryName);
}
return queryToRun;
}
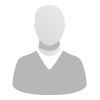
Hi Brandy,
if you have missing code pieces, try searching the blog e.g. for projectArea. A lot of the code uses the same infrastructure. Links to this are also provided: e.g. the wiki entry on Programmatic Work Item Creation or https://jazz.net/wiki/bin/view/Main/QueryDevGuide in https://rsjazz.wordpress.com/2012/10/29/using-work-item-queris-for-automation/
Unfortunately I can not package all the code and sometimes the blog software also interferes with the code.
if you have missing code pieces, try searching the blog e.g. for projectArea. A lot of the code uses the same infrastructure. Links to this are also provided: e.g. the wiki entry on Programmatic Work Item Creation or https://jazz.net/wiki/bin/view/Main/QueryDevGuide in https://rsjazz.wordpress.com/2012/10/29/using-work-item-queris-for-automation/
Unfortunately I can not package all the code and sometimes the blog software also interferes with the code.