Disallow few combinations of an Enum List
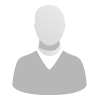
Accepted answer
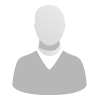
var enumList = workItem.getValue("enumList");
var enumSet = new Set();
for (var i=0; i<enumList.length; i++) {
enumSet.add(enumList[i].label);
}
console.log("enumSet: " + enumSet);
if (enumSet.has("A") && enumSet.size>1) {
return new Status(Severity["ERROR"], "When A is chosen, no other values are allowed");
} else if (enumSet.has("B") && enumSet.has("C") && enumSet.has("D") && enumSet.has("E")) {
return new Status(Severity["ERROR"], "B, C, D&E are not allowed as a combination");
} else {
return Status.OK_STATUS;
}
Comments
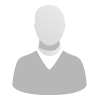
Thanks for the detailed explanation and the script.
Where will console.log write to; is it the Tomcat console that opens at the time of starting the application ?
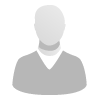
Depending on which client you are using, but it will not appear in the Tomcat console or the catalina.out file.
If you are using RTC Eclipse client, the message will appear in the .metadata/.log file under the Eclipse workspace.
If you are using a browser, the message will appear in the web console.
If the script is executed on the server side, the message will appear in the .metadata/.log file under the CCM Eclipse workspace (for example, $JAZZ_HOME/server/tomcat/work/Catalina/localhost/ccm/eclipse/workspace/.metadata/.log). Note that execution errors will appear in ccm.log as well so you need to check this file when debugging the script.
One other answer
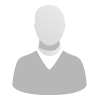
Comments
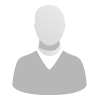
By tree view value set, I believe you are referring to Dependent Enum, where 2 Enum attributes will be defined.
In my requirement, one enum att is only allowed. One rule states that maximum of 3 values can be choosen.
Can you also guide me on how to access the attribute value of an Enum list in dojo script.

Yes, that is correct, dependant enum..
I do not know how to use dojo for this